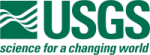 |
Isis 3 Programmer Reference
|
10 #include "ShapeModel.h"
12 template<
class T>
class QVector;
64 using Isis::ShapeModel::intersectSurface;
68 std::vector<double> lookDirection);
DemShape()
Construct a DemShape object.
double demScale()
Return the scale of the DEM shape, in pixels per degree.
Buffer for containing a two dimensional section of an image.
This class is designed to encapsulate the concept of a Latitude.
bool intersectSurface(std::vector< double > observerPos, std::vector< double > lookDirection)
Find the intersection point with the DEM.
Projection * m_demProj
The projection of the model.
Cube * demCube()
Returns the cube defining the shape model.
Portal * m_portal
Buffer used to read from the model.
Container for cube-like labels.
virtual void calculateDefaultNormal()
This method calculates the default normal (Ellipsoid for backwards compatability) for the DemShape.
void calculateLocalNormal(QVector< double * > cornerNeighborPoints)
This method calculates the local surface normal of the current intersection point.
Distance measurement, usually in meters.
This class is designed to encapsulate the concept of a Longitude.
Distance localRadius(const Latitude &lat, const Longitude &lon)
Gets the radius from the DEM, if we have one.
~DemShape()
Destroys the DemShape.
Interpolator * m_interp
Use bilinear interpolation from dem.
double m_pixPerDegree
Scale of DEM file in pixels per degree.
IO Handler for Isis Cubes.
Define shapes and provide utilities for targets stored as ISIS maps.
Define shapes and provide utilities for Isis targets.
This is free and unencumbered software released into the public domain.
Base class for Map Projections.
This class is used to create and store valid Isis targets.
bool isDEM() const
Indicates that this shape model is from a DEM.
void calculateSurfaceNormal()
This method calculates the surface normal of the current intersection point.
This is free and unencumbered software released into the public domain.
Cube * m_demCube
The cube containing the model.