Loading [MathJax]/jax/output/NativeMML/config.js
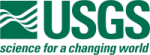 |
Isis 3 Programmer Reference
|
27 #include "CubeManager.h"
29 #include "EllipsoidShape.h"
31 #include "IException.h"
32 #include "Interpolator.h"
35 #include "Longitude.h"
36 #include "NaifStatus.h"
38 #include "Projection.h"
41 #include "SurfacePoint.h"
44 #include "UniqueIOCachingAlgorithm.h"
82 demCubeFile = (QString) kernels[
"ElevationModel"];
85 demCubeFile = (QString) kernels[
"ShapeModel"];
144 vector<double> lookDirection) {
150 if (!ellipseIntersected) {
155 static const int maxit = 100;
157 double dX, dY, dZ, dist2;
165 SpiceDouble currentIntersectPt[3];
166 SpiceDouble newIntersectPt[3];
174 double tol2 = tol * tol;
188 double t = newIntersectPt[0] * newIntersectPt[0] +
189 newIntersectPt[1] * newIntersectPt[1];
191 latDD = atan2(newIntersectPt[2], sqrt(t)) *
RAD2DEG;
192 lonDD = atan2(newIntersectPt[1], newIntersectPt[0]) *
RAD2DEG;
210 memcpy(currentIntersectPt, newIntersectPt, 3 *
sizeof(
double));
214 surfpt_c((SpiceDouble *) &observerPos[0], &lookDirection[0], r, r, r, newIntersectPt,
215 (SpiceBoolean*) &status);
232 dX = currentIntersectPt[0] - newIntersectPt[0];
233 dY = currentIntersectPt[1] - newIntersectPt[1];
234 dZ = currentIntersectPt[2] - newIntersectPt[2];
235 dist2 = (dX*dX + dY*dY + dZ*dZ) * 1000 * 1000;
308 IString msg =
"A valid intersection must be defined before computing the surface normal";
320 double a = radii[0].kilometers();
321 double b = radii[1].kilometers();
322 double c = radii[2].kilometers();
324 vector<double>
normal(3,0.);
327 surfnm_c(a, b, c, pB, (SpiceDouble *) &
normal[0]);
369 std::vector<SpiceDouble>
normal(3);
370 if (neighborPoints.isEmpty()) {
378 double topMinusBottom[3];
379 vsub_c(neighborPoints[0], neighborPoints[1], topMinusBottom);
380 double rightMinusLeft[3];
381 vsub_c(neighborPoints[3], neighborPoints [2], rightMinusLeft);
384 ucrss_c(topMinusBottom, rightMinusLeft, (SpiceDouble *) &
normal[0]);
388 unorm_c((SpiceDouble *) &
normal[0], (SpiceDouble *) &
normal[0], &mag);
404 double centerLookVect[3];
407 unorm_c(pB, centerLookVect, &mag);
408 double dotprod = vdot_c((SpiceDouble *) &
normal[0], centerLookVect);
410 vminus_c((SpiceDouble *) &
normal[0], (SpiceDouble *) &
normal[0]);
DemShape()
Construct a DemShape object.
double kilometers() const
Get the distance in kilometers.
@ Degrees
Degrees are generally considered more human readable, 0-360 is one circle, however most math does not...
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
double demScale()
Return the scale of the DEM shape, in pixels per degree.
Buffer for containing a two dimensional section of an image.
This class is designed to encapsulate the concept of a Latitude.
bool intersectSurface(std::vector< double > observerPos, std::vector< double > lookDirection)
Find the intersection point with the DEM.
SurfacePoint * surfaceIntersection() const
Returns the surface intersection for this ShapeModel.
Projection * m_demProj
The projection of the model.
void read(Blob &blob, const std::vector< PvlKeyword > keywords=std::vector< PvlKeyword >()) const
This method will read data from the specified Blob object.
std::vector< Distance > targetRadii() const
Returns the radii of the body in km.
void setHasIntersection(bool b)
Sets the flag to indicate whether this ShapeModel has an intersection.
double * DoubleBuffer() const
Returns the value of the shape buffer.
Cube * demCube()
Returns the cube defining the shape model.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Portal * m_portal
Buffer used to read from the model.
Container for cube-like labels.
int Lines()
Returns the number of lines needed by the interpolator.
virtual void calculateDefaultNormal()
This method calculates the default normal (Ellipsoid for backwards compatability) for the DemShape.
static Cube * Open(const QString &cubeFileName)
This method calls the method OpenCube() on the static instance.
virtual bool SetUniversalGround(const double coord1, const double coord2)
This method is used to set the lat/lon or radius/azimuth (i.e.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
void setNormal(const std::vector< double >)
Sets the normal for the currect intersection point.
bool IsSpecial(const double d)
Returns if the input pixel is special.
double HotSample()
Returns the sample coordinate of the center pixel in the buffer for the interpolator.
void calculateLocalNormal(QVector< double * > cornerNeighborPoints)
This method calculates the local surface normal of the current intersection point.
Distance measurement, usually in meters.
This class is designed to encapsulate the concept of a Longitude.
@ Traverse
Search child objects.
Distance localRadius(const Latitude &lat, const Longitude &lon)
Gets the radius from the DEM, if we have one.
virtual double WorldY() const
This returns the world Y coordinate provided SetGround, SetCoordinate, SetUniversalGround,...
void setName(QString name)
Sets the shape name.
double resolution()
Convenience method to get pixel resolution (m/pix) at current intersection point.
~DemShape()
Destroys the DemShape.
@ Meters
The distance is being specified in meters.
Interpolator * m_interp
Use bilinear interpolation from dem.
Contains multiple PvlContainers.
void addCachingAlgorithm(CubeCachingAlgorithm *)
This will add the given caching algorithm to the list of attempted caching algorithms.
double m_pixPerDegree
Scale of DEM file in pixels per degree.
bool intersectEllipsoid(const std::vector< double > observerPosRelativeToTarget, const std::vector< double > &observerLookVectorToTarget)
Finds the intersection point on the ellipsoid model using the given position of the observer (spacecr...
virtual double WorldX() const
This returns the world X coordinate provided SetGround, SetCoordinate, SetUniversalGround,...
void FromNaifArray(const double naifValues[3])
A naif array is a c-style array of size 3.
IO Handler for Isis Cubes.
void setHasNormal(bool status)
Sets the flag to indicate whether this ShapeModel has a surface normal.
double kilometers() const
Get the displacement in kilometers.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
Define shapes and provide utilities for Isis targets.
bool isValid() const
This indicates whether we have a legitimate angle stored or are in an unset, or invalid,...
PixelType pixelType() const
double Interpolate(const double isamp, const double iline, const double buf[])
Performs an interpolation on the data according to the parameters set in the constructor.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
void ToNaifArray(double naifOutput[3]) const
A naif array is a c-style array of size 3.
This algorithm is designed for applications that jump around between a couple of spots in the cube wi...
double degrees() const
Get the angle in units of Degrees.
bool hasIntersection()
Returns intersection status.
int Samples()
Returns the number of samples needed by the interpolator.
Adds specific functionality to C++ strings.
This is free and unencumbered software released into the public domain.
double HotLine()
Returns the line coordinate of the center pixel in the buffer for the interpolator.
void SetPosition(const double sample, const double line, const int band)
Sets the line and sample position of the buffer.
This class is used to create and store valid Isis targets.
const double RAD2DEG
Multiplier for converting from radians to degrees.
Projection * projection()
bool isDEM() const
Indicates that this shape model is from a DEM.
void calculateSurfaceNormal()
This method calculates the surface normal of the current intersection point.
This is free and unencumbered software released into the public domain.
Cube * m_demCube
The cube containing the model.
std::vector< double > normal()
Returns the local surface normal at the current intersection point.