File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
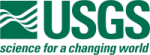 |
Isis 3 Programmer Reference
|
9 #include "LroWideAngleCamera.h"
10 #include "LroWideAngleCameraFocalPlaneMap.h"
11 #include "LroWideAngleCameraDistortionMap.h"
19 #include "CameraFocalPlaneMap.h"
20 #include "CameraSkyMap.h"
21 #include "CollectorMap.h"
22 #include "IException.h"
25 #include "NaifStatus.h"
26 #include "PushFrameCameraDetectorMap.h"
27 #include "PushFrameCameraGroundMap.h"
41 LroWideAngleCamera::LroWideAngleCamera(
Cube &cube) :
59 QString stime = inst[
"SpacecraftClockStartCount"];
68 QString instId = inst[
"InstrumentId"][0].toUpper();
70 int frameletSize = 14;
72 int filterIKBase = 10;
74 if (instId ==
"WAC-UV") {
77 filterIKBase = 15 - 1;
81 else if (instId ==
"WAC-VIS") {
84 filterIKBase = 10 - 3;
89 QString msg =
"Invalid value [" + instId
90 +
"] for keyword [InstrumentId]";
99 const PvlKeyword &filtNames = bandBin[
"Center"];
102 if (nbands != filtNames.
size()) {
104 mess <<
"Number bands in (file) label (" << nbands
105 <<
") do not match number of values in BandBin/Center keyword ("
106 << filtNames.
size() <<
") - required for band-dependent geoemtry";
111 bool dataflipped = (inst[
"DataFlipped"][0].toUpper() ==
"YES");
114 QString instCode =
"INS" + QString::number(
naifIkCode());
116 QString ikernKey = instCode +
"_FILTER_BANDCENTER";
119 ikernKey = instCode +
"_FILTER_OFFSET";
123 ikernKey = instCode +
"_FILTER_BANDID";
131 for (
int i = 0 ; i < foffset.size() ; i++) {
132 filterToDetectorOffset.
add(fbc[i], foffset[i]);
133 wavel.
add(foffset[i], fbc[i]);
134 filterIKCode.
add(fbc[i],
naifIkCode() - (filterIKBase + fbandid[i]));
140 int frameletOffsetFactor = inst[
"ColorOffset"];
141 if ( dataflipped ) frameletOffsetFactor *= -1;
143 for (
int j = 0 ; j < wavel.
size() ; j++) {
144 int wavelen = wavel.
getNth(j);
145 filterToFrameletOffset.
add(wavelen, j * frameletOffsetFactor);
150 for (
int i = 0; i < filtNames.
size(); i++) {
151 if (!filterToDetectorOffset.
exists(filtNames[i].toInt())) {
152 QString msg =
"Unrecognized filter name [" + filtNames[i] +
"]";
156 p_detectorStartLines.push_back(filterToDetectorOffset.
get(filtNames[i].toInt()));
157 p_frameletOffsets.push_back(filterToFrameletOffset.
get(filtNames[i].toInt()));
159 QString kBase =
"INS" + QString::number(filterIKCode.
get(filtNames[i].toInt()));
160 p_focalLength.push_back(
getDouble(kBase+
"_FOCAL_LENGTH"));
161 p_boreSightSample.push_back(
getDouble(kBase+
"_BORESIGHT_SAMPLE"));
162 p_boreSightLine.push_back(
getDouble(kBase+
"_BORESIGHT_LINE"));
166 double frameletRate = (double) inst[
"InterframeDelay"] / 1000.0;
173 bool flippedFramelets = dataflipped;
178 QString instModeId = inst[
"InstrumentModeId"][0].toUpper();
181 if (instModeId ==
"BW") {
182 instModeId += inst[
"Mode"][0];
185 p_frameletOffsets[0] = 0;
188 ikernKey = instCode +
"_" + instModeId +
"_SAMPLE_OFFSET";
195 for (
int i = 0 ; i < filtNames.
size() ; i++ ) {
196 fplane->
addFilter(filterIKCode.
get(filtNames[i].toInt()));
197 distort->
addFilter(filterIKCode.
get(filtNames[i].toInt()));
201 bool evenFramelets = (inst[
"Framelets"][0].toUpper() ==
"EVEN");
209 if(instId ==
"WAC-UV") {
234 int maxbands = min(p_detectorStartLines.size(), p_frameletOffsets.size());
235 if ((vband <= 0) || (vband > maxbands)) {
237 mess <<
"Requested virtual band (" << vband
238 <<
") outside valid (BandBin/Center) limits (1 - " << maxbands
255 p_boreSightLine[vband-1] + 1.0);
272 dtpool_c(key.toLatin1().data(), &found, &n, ctype);
284 QVariant poolKeySize = getStoredResult(key +
"_SIZE",
SpiceIntType);
286 int nvals = poolKeySize.toInt();
294 QString mess =
"Kernel pool keyword " + key +
" not found!";
299 for (
int i = 0 ; i < nvals ; i++) {
@ SpiceIntType
SpiceInt type.
virtual void SetBand(const int band)
Virtual method that sets the band number.
IntParameterList GetVector(const QString &key)
void SetDetectorOrigin(const double sample, const double line)
Set the detector origin.
void SetFrameletOffset(int frameletOffset)
Reset the frame offset.
void SetGeometricTilingHint(int startSize=128, int endSize=8)
This method sets the best geometric tiling size for projecting from this camera model.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
virtual int CkFrameId() const
CK frame ID - - Instrument Code from spacit run on CK.
int ParentLines() const
Returns the number of lines in the parent alphacube.
A single keyword-value pair.
~LroWideAngleCamera()
Destroys the LroWideAngleCamera object.
T & getNth(int nth)
Returns the nth value in the collection.
SpiceInt naifIkCode() const
This returns the NAIF IK code to use when reading from instrument kernels.
void SetFrameletOrderReversed(bool frameletOrderReversed, int nframelets)
Changes the direction of the framelets.
void SetFrameletsGeometricallyFlipped(bool frameletsFlipped)
Mirrors the each framelet in the file.
QString m_instrumentNameLong
Full instrument name.
virtual iTime getClockTime(QString clockValue, int sclkCode=-1, bool clockTicks=false)
This converts the spacecraft clock ticks value (clockValue) to an iTime.
T & get(const K &key)
Returns the value associated with the name provided.
Distort/undistort focal plane coordinates.
void SetDetectorSampleSumming(const double summing)
Set sample summing mode.
int size() const
Returns the size of the collection.
SpiceInt getInteger(const QString &key, int index=0)
This returns a value from the NAIF text pool.
Container for cube-like labels.
CameraDistortionMap * DistortionMap()
Returns a pointer to the CameraDistortionMap object.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
bool IsBandIndependent()
The camera model is band dependent, so this method returns false.
double p_exposureDur
Exposure Duration value from labels.
virtual SpiceRotation * instrumentRotation() const
Accessor method for the instrument rotation.
void SetDetectorLineSumming(const double summing)
Set line summing mode.
@ Traverse
Search child objects.
PushFrameCameraDetectorMap * DetectorMap()
Returns a pointer to the PushFrameCameraDetectorMap object.
bool exists(const K &key) const
Checks the existance of a particular key in the list.
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
void SetPixelPitch()
Reads the Pixel Pitch from the instrument kernel.
Contains multiple PvlContainers.
void addFilter(int naifIkCode)
Add an additional set of parameters for a given LROC/WAC filter.
double p_etStart
Ephemeris Start iTime.
virtual int SpkReferenceId() const
SPK Reference ID - J2000.
void setBand(int vband)
Implements band-dependant distortion parameters.
Distort/undistort focal plane coordinates.
int PoolKeySize(const QString &key) const
void setBand(int vband)
Implements band-dependant focal plane parameters.
Generic class for Push Frame Cameras.
IO Handler for Isis Cubes.
QString m_spacecraftNameLong
Full spacecraft name.
void SetStartingDetectorSample(const double sample)
Set the starting detector sample.
void add(const K &key, const T &value)
Adds the element to the list.
double toDouble(const QString &string)
Global function to convert from a string to a double.
CameraFocalPlaneMap * FocalPlaneMap()
Returns a pointer to the CameraFocalPlaneMap object.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
void LoadCache()
This loads the spice cache big enough for this image.
QString m_instrumentNameShort
Shortened instrument name.
Collector/container for arbitrary items.
void SetBand(const int band)
Sets the band in the camera model.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
Convert between undistorted focal plane and ra/dec coordinates.
void SetBandFirstDetectorLine(int firstLine)
Change the starting line in the detector based on band.
int size() const
Returns the number of values stored in this keyword.
LRO Wide Angle Camera Model.
void addFilter(int naifIkCode)
Add an additional set of parameters for a given LROC/WAC filter.
PvlKeyword & findKeyword(const QString &kname, FindOptions opts)
Finds a keyword in the current PvlObject, or deeper inside other PvlObjects and Pvlgroups within this...
virtual int CkReferenceId() const
CK Reference ID - J2000.
SpiceDouble getDouble(const QString &key, int index=0)
This returns a value from the NAIF text pool.
void SetFrame(int frameCode)
Change the frame to the given frame code.
Convert between undistorted focal plane and ground coordinates.
QString m_spacecraftNameShort
Shortened spacecraft name.
Convert between parent image coordinates and detector coordinates.
void SetFocalLength()
Reads the focal length from the instrument kernel.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
int p_nframelets
Number of framelets in whole image.