Loading [MathJax]/jax/output/NativeMML/config.js
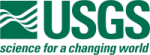 |
Isis 3 Programmer Reference
|
7 #include "PushFrameCameraGroundMap.h"
11 #include "CameraDistortionMap.h"
12 #include "CameraFocalPlaneMap.h"
15 #include "Longitude.h"
16 #include "PushFrameCameraDetectorMap.h"
17 #include "SurfacePoint.h"
35 int startFramelet = 1;
41 bool minimizedSpacecraftDist =
false;
43 for (
int j = 0; j < 30 && !minimizedSpacecraftDist;j++) {
44 int deltaX = abs(startFramelet - endFramelet) / 2;
53 double biasFactor = startDist / endDist;
55 if (biasFactor < 1.0) {
56 biasFactor = -1.0 / biasFactor;
57 biasFactor = -(biasFactor + 1) / biasFactor;
60 biasFactor = std::min(biasFactor + 0.50, 0.0);
63 biasFactor = (biasFactor - 1) / biasFactor;
66 biasFactor = std::max(biasFactor - 0.50, 0.0);
69 int middleFramelet = startFramelet + (int)(deltaX + biasFactor * deltaX);
72 if (startDist > endDist) {
74 if (startFramelet == middleFramelet) middleFramelet++;
75 startFramelet = middleFramelet;
76 startDist = middleDist;
79 endFramelet = middleFramelet;
83 if (startFramelet == endFramelet) {
84 minimizedSpacecraftDist =
true;
88 if (!minimizedSpacecraftDist) {
92 int realFramelet = startFramelet;
93 bool frameletEven = (realFramelet % 2 == 0);
104 double realDist =
FindDistance(realFramelet, surfacePoint);
105 int guessFramelet = realFramelet + direction;
106 double guessDist =
FindDistance(guessFramelet, surfacePoint);
108 if (guessDist > realDist) {
109 direction = -1 * direction;
110 guessFramelet = realFramelet + direction;
114 for (
int j = 0; (realDist >= guessDist) && (j < 30);j++) {
115 realFramelet = guessFramelet;
116 realDist = guessDist;
118 guessFramelet = realFramelet + direction;
121 if (realFramelet <= 0 || realFramelet > detectorMap->
TotalFramelets()) {
153 if(!
p_camera->Sensor::SetGround(surfacePoint,
false))
return DBL_MAX;
156 p_camera->Sensor::LookDirection(lookC);
171 double frameletDeltaY = detectorMap->
frameletLine() - (actualFrameletHeight / 2.0);
173 return frameletDeltaY * frameletDeltaY;
191 if(!
p_camera->Sensor::SetGround(surfacePoint,
false))
return DBL_MAX;
double FindDistance(int framelet, const SurfacePoint &surfacePoint)
This method finds the distance from the center of the framelet to the lat,lon.
This class is designed to encapsulate the concept of a Latitude.
int TotalFramelets() const
Return the total number of framelets including padding.
void SetFramelet(int framelet, const double deltaT=0)
This method changes the current framelet.
virtual bool SetUndistortedFocalPlane(double ux, double uy)
Compute distorted focal plane x/y.
double FocalPlaneX() const
Gets the x-value in the focal plane coordinate system.
double DetectorLine() const
double FocalPlaneY() const
Gets the y-value in the focal plane coordinate system.
CameraDistortionMap * DistortionMap()
Returns a pointer to the CameraDistortionMap object.
Latitude GetLatitude() const
Return the body-fixed latitude for the surface point.
double DetectorSample() const
virtual bool SetGround(const Latitude &lat, const Longitude &lon)
Compute undistorted focal plane coordinate from ground position.
This class is designed to encapsulate the concept of a Longitude.
int frameletHeight() const
This returns how many lines are considered a single framelet.
virtual bool SetFocalPlane(const double dx, const double dy)
Compute detector position (sample,line) from focal plane coordinates.
virtual bool SetDetector(const double sample, const double line)
Compute parent position from a detector coordinate.
double frameletLine() const
This returns the calculated framelet line.
Distort/undistort focal plane coordinates.
virtual double LineScaleFactor() const
Return scaling factor for computing line resolution.
Longitude GetLongitude() const
Return the body-fixed longitude for the surface point.
CameraFocalPlaneMap * FocalPlaneMap()
Returns a pointer to the CameraFocalPlaneMap object.
double FocalLength() const
Returns the focal length.
Convert between distorted focal plane and detector coordinates.
CameraDetectorMap * DetectorMap()
Returns a pointer to the CameraDetectorMap object.
double FindSpacecraftDistance(int framelet, const SurfacePoint &surfacePoint)
This method finds the distance from the point on the ground to the spacecraft at the time the specifi...
Convert between parent image coordinates and detector coordinates.
This class defines a body-fixed surface point.
This is free and unencumbered software released into the public domain.
virtual double SlantDistance() const
Return the distance between the spacecraft and surface point in kmv.
Distance LocalRadius() const
Returns the local radius at the intersection point.
bool p_evenFramelets
True if the file contains even framelets.
virtual bool SetGround(const Latitude &lat, const Longitude &lon)
Compute undistorted focal plane coordinate from ground position.
bool timeAscendingFramelets()
Returns if the framelets are reversed from top-to-bottom.