Loading [MathJax]/jax/output/NativeMML/config.js
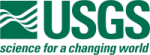 |
Isis 3 Programmer Reference
|
9 #include "CsmBundleObservation.h"
13 #include <QStringList>
16 #include "BundleImage.h"
17 #include "BundleControlPoint.h"
18 #include "BundleObservationSolveSettings.h"
19 #include "BundleTargetBody.h"
21 #include "CSMCamera.h"
22 #include "LinearAlgebra.h"
23 #include "SpicePosition.h"
24 #include "SpiceRotation.h"
33 CsmBundleObservation::CsmBundleObservation() {
46 CsmBundleObservation::CsmBundleObservation(
BundleImageQsp image, QString observationNumber,
48 if (bundleTargetBody) {
49 QString msg =
"Target body parameters cannot be solved for with CSM observations.";
108 m_corrections.clear();
127 m_corrections.resize(nParams);
131 for (
int i = 0; i < nParams; i++) {
166 QString msg =
"Invalid correction vector passed to observation.";
172 for (
size_t i = 0; i < corrections.size(); i++) {
177 m_corrections += corrections;
217 for (
int i = 0; i < nParameters; i++) {
226 QString adjustedSigma;
229 for (
int i = 0; i < nParameters; i++) {
231 correction = m_corrections(i);
235 sprintf(buf,
"%.11s", parameterNamesList.at(i).toStdString().c_str());
237 sprintf(buf,
"%18.8lf ", finalParameterValues[i] - correction);
239 sprintf(buf,
"%20.8lf ", correction);
241 sprintf(buf,
"%23.8lf ", finalParameterValues[i]);
245 sprintf(buf,
"%6s", sigma.toStdString().c_str());
249 if (errorPropagation) {
250 sprintf(buf,
"%s", adjustedSigma.toStdString().c_str());
253 sprintf(buf,
"%s",
"N/A");
258 sprintf(buf,
"%s\n", parameterUnitList.at(i).toStdString().c_str());
275 QString finalqStr =
"";
280 finalqStr +=
toString(finalValue - m_corrections[i]) +
",";
281 finalqStr +=
toString(m_corrections[i]) +
",";
282 finalqStr +=
toString(finalValue) +
",";
284 if (errorPropagation) {
330 if (bundleTargetBody) {
331 QString msg =
"Target body parameters cannot be solved for with CSM observations.";
358 vector<double> partials = csmCamera->getSensorPartials(
m_paramIndices[i], groundPoint);
359 coeffImage(0, i) = partials[1];
360 coeffImage(1, i) = partials[0];
382 coeffPoint3D.clear();
388 vector<double> groundPartials = measureCamera->
GroundPartials(groundPoint);
399 coeffPoint3D(1,0) = groundPartials[0] * 1000;
400 coeffPoint3D(1,1) = groundPartials[1] * 1000;
401 coeffPoint3D(1,2) = groundPartials[2] * 1000;
402 coeffPoint3D(0,0) = groundPartials[3] * 1000;
403 coeffPoint3D(0,1) = groundPartials[4] * 1000;
404 coeffPoint3D(0,2) = groundPartials[5] * 1000;
412 coeffPoint3D(1,0) = 1000 * (groundPartials[0]*latDerivative[0] + groundPartials[1]*latDerivative[1] + groundPartials[2]*latDerivative[2]);
413 coeffPoint3D(1,1) = 1000 * (groundPartials[0]*lonDerivative[0] + groundPartials[1]*lonDerivative[1] + groundPartials[2]*lonDerivative[2]);
414 coeffPoint3D(1,2) = 1000 * (groundPartials[0]*radDerivative[0] + groundPartials[1]*radDerivative[1] + groundPartials[2]*radDerivative[2]);
417 coeffPoint3D(0,0) = 1000 * (groundPartials[3]*latDerivative[0] + groundPartials[4]*latDerivative[1] + groundPartials[5]*latDerivative[2]);
418 coeffPoint3D(0,1) = 1000 * (groundPartials[3]*lonDerivative[0] + groundPartials[4]*lonDerivative[1] + groundPartials[5]*lonDerivative[2]);
419 coeffPoint3D(0,2) = 1000 * (groundPartials[3]*radDerivative[0] + groundPartials[4]*radDerivative[1] + groundPartials[5]*radDerivative[2]);
422 IString msg =
"Unknown surface point coordinate type enum [" +
toString(coordType) +
"]." ;
450 QString msg =
"Unable to map apriori surface point for measure ";
454 double computedSample = measureCamera->
Sample();
455 double computedLine = measureCamera->
Line();
459 double deltaSample = measure.
sample() - computedSample;
460 double deltaLine = measure.
line() - computedLine;
462 coeffRHS(0) = deltaSample;
463 coeffRHS(1) = deltaLine;
@ Type
Solve for all CSM parameters of a specific type.
double getParameterCovariance(int index1, int index2)
Get the covariance between two parameters.
QString cubeSerialNumber() const
Accesses the serial number of the cube containing this control measure.
SurfacePoint adjustedSurfacePoint() const
Accesses the adjusted SurfacePoint associated with this BundleControlPoint.
@ Set
Solve for all CSM parameters belonging to a specific set.
bool computeImagePartials(LinearAlgebra::Matrix &coeffImage, BundleMeasure &measure)
Calculates the sensor partials with respect to the solve parameters and populates the coeffImage matr...
virtual double Sample() const
Returns the current sample number.
void bundleOutputString(std::ostream &fpOut, bool errorPropagation)
Takes in an open std::ofstream and writes out information which goes into the bundleout....
void applyParameterCorrection(int index, double correction)
Adjust the value of a parameter.
Camera * camera() const
Accesses the associated camera for this bundle measure.
This class holds information about a control point that BundleAdjust needs to run correctly.
virtual bool SetGround(Latitude latitude, Longitude longitude)
Sets the lat/lon values to get the sample/line values.
boost::numeric::ublas::matrix< double > Matrix
Definition for an Isis::LinearAlgebra::Matrix of doubles.
LinearAlgebra::Vector m_weights
Parameter weights. Cumulative parameter correction vector.
QString id() const
Accesses the Point ID associated with this BundleControlPoint.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
Class for observations that use CSM camera models in bundle adjustment.
bool IsSpecial(const double d)
Returns if the input pixel is special.
QString bundleOutputCSV(bool errorPropagation)
Creates and returns a formatted QString representing the bundle coefficients and parameters in csv fo...
std::vector< double > LatitudinalDerivative(CoordIndex index)
Compute partial derivative of the conversion of the latitudinal coordinates to body-fixed rectangular...
QSharedPointer< BundleObservationSolveSettings > BundleObservationSolveSettingsQsp
Definition for BundleObservationSolveSettingsQsp, a QSharedPointer to a BundleObservationSolveSetting...
double getParameterValue(int index)
Get the value of a parameter.
LinearAlgebra::Vector m_aprioriSigmas
A posteriori (adjusted) parameter sigmas.
bool computePoint3DPartials(LinearAlgebra::Matrix &coeffPoint3D, BundleMeasure &measure, SurfacePoint::CoordinateType coordType)
Calculates the ground partials for the line, sample currently set in the sensor model.
~CsmBundleObservation()
Destructor.
@ Rectangular
Body-fixed rectangular x/y/z coordinates.
boost::numeric::ublas::vector< double > Vector
Definition for an Isis::LinearAlgebra::Vector of doubles.
CoordinateType
Defines the coordinate typ, units, and coordinate index for some of the output methods.
BundleControlPoint * parentControlPoint()
Accesses the parent BundleControlPoint for this bundle measure.
double computeObservationValue(BundleMeasure &measure, double deltaVal)
Returns the observed value in (sample, line) coordinates.
double sample() const
Accesses the current sample measurement for this control measure.
bool computeTargetPartials(LinearAlgebra::Matrix &coeffTarget, BundleMeasure &measure, BundleSettingsQsp &bundleSettings, BundleTargetBodyQsp &bundleTargetBody)
Cannot compute target body parameters for a CSM observation, so always throws an exception.
virtual bool setSolveSettings(BundleObservationSolveSettings solveSettings)
Set solve parameters.
CsmBundleObservation & operator=(const CsmBundleObservation &src)
Assignment operator.
A container class for a ControlMeasure.
bool computeRHSPartials(LinearAlgebra::Vector &coeffRHS, BundleMeasure &measure)
Calculates the sample, line residuals between the values measured in the image and the ground-to-imag...
const BundleObservationSolveSettingsQsp solveSettings()
Accesses the solve settings.
std::vector< int > getParameterIndices(csm::param::Set paramSet) const
Get the indices of the parameters that belong to a set.
BundleObservationSolveSettingsQsp m_solveSettings
Solve settings for this observation.
bool applyParameterCorrections(LinearAlgebra::Vector corrections)
Applies the parameter corrections.
@ Programmer
This error is for when a programmer made an API call that was illegal.
QString getParameterUnits(int index)
Get the units of the parameter at a particular index.
Namespace for the standard library.
QString getParameterName(int index)
Get the name of the parameter.
CsmBundleObservation()
Constructs a CsmBundleObservation initialized to a default state.
LinearAlgebra::Vector m_adjustedSigmas
A posteriori (adjusted) parameter sigmas.
@ List
Solve for an explicit list of CSM parameters.
This class is used to modify and manage solve settings for 1 to many BundleObservations.
@ Latitudinal
Planetocentric latitudinal (lat/lon/rad) coordinates.
Adds specific functionality to C++ strings.
virtual double Line() const
Returns the current line number.
virtual std::vector< double > GroundPartials(SurfacePoint groundPoint)
Compute the partial derivatives of the sample, line with respect to the x, y, z coordinates of the gr...
std::vector< int > m_paramIndices
The indices of the parameters the observation is solving for.
double line() const
Accesses the current line measurement for this control measure.
This class defines a body-fixed surface point.
This is free and unencumbered software released into the public domain.
int numberParameters()
Returns the number of total parameters there are for solving.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
Abstract base class for an observation in bundle adjustment.
virtual QStringList parameterList()
Returns the list of observation parameter names.