File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
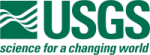 |
Isis 3 Programmer Reference
|
9 #include "BundleControlPoint.h"
14 #include <boost/numeric/ublas/vector_proxy.hpp>
17 #include "ControlMeasure.h"
19 #include "LinearAlgebra.h"
20 #include "Longitude.h"
21 #include "SparseBlockMatrix.h"
22 #include "SpecialPixel.h"
35 m_controlPoint = controlPoint;
38 int numMeasures = controlPoint->GetNumMeasures();
39 for (
int i = 0; i < numMeasures; i++) {
41 if (controlMeasure->IsIgnored()) {
66 m_coordTypeBundle = bundleSettings->controlPointCoordTypeBundle();
99 m_controlPoint = src.m_controlPoint;
101 int numMeasures = src.size();
103 for (
int i = 0; i < numMeasures; i++)
112 m_coordTypeBundle = src.m_coordTypeBundle;
129 append(bundleMeasure);
131 return bundleMeasure;
188 double globalPointCoord1AprioriSigma = settings->globalPointCoord1AprioriSigma();
189 double globalPointCoord2AprioriSigma = settings->globalPointCoord2AprioriSigma();
190 double globalPointCoord3AprioriSigma = settings->globalPointCoord3AprioriSigma();
204 if (!
IsSpecial(globalPointCoord1AprioriSigma)) {
208 if (!
IsSpecial(globalPointCoord2AprioriSigma)) {
217 if (!
IsSpecial(globalPointCoord3AprioriSigma)) {
234 m_controlPoint->GetAprioriSurfacePoint().
GetWeight(m_coordTypeBundle, SurfacePoint::One);
236 else if (!
IsSpecial(globalPointCoord1AprioriSigma)) {
246 m_controlPoint->GetAprioriSurfacePoint().
GetWeight(m_coordTypeBundle, SurfacePoint::Two);
248 else if (!
IsSpecial(globalPointCoord2AprioriSigma)) {
262 (m_coordTypeBundle, SurfacePoint::Three);
264 else if (!
IsSpecial(globalPointCoord3AprioriSigma)) {
290 double sigmaRadians =
292 if (sigmaRadians > DBL_EPSILON) {
293 m_weights[index] = 1. / (sigmaRadians*sigmaRadians);
297 double sigmakm = gSigma * .001;
298 if (sigmakm > DBL_EPSILON) {
303 IString msg =
"Unknown surface point coordinate type enum ["
304 +
toString(m_coordTypeBundle) +
"]." ;
311 double sigmaRadians =
313 if (sigmaRadians > DBL_EPSILON) {
314 m_weights[1] = 1. / (sigmaRadians*sigmaRadians);
318 double sigmakm = gSigma * 0.001;
319 if (sigmakm > DBL_EPSILON)
m_weights[1] = 1./ (sigmakm*sigmakm);
322 IString msg =
"Unknown surface point coordinate type enum ["
323 +
toString(m_coordTypeBundle) +
"]." ;
331 double sigmakm = gSigma * .001;
368 int subrangeStart, subrangeEnd;
370 while ( Qit.hasNext() ) {
373 int columnIndex = Qit.key();
375 subrangeStart = sparseNormals.at(columnIndex)->startColumn();
376 subrangeEnd = subrangeStart + Qit.value()->size2();
378 m_nicVector += alpha * prod(*(Qit.value()),subrange(v1,subrangeStart,subrangeEnd));
399 switch (m_coordTypeBundle) {
407 IString msg =
"Unknown surface point coordinate type enum ["
408 +
toString(m_coordTypeBundle) +
"]." ;
426 return m_controlPoint;
436 return m_controlPoint->IsRejected();
480 return m_controlPoint->GetAdjustedSurfacePoint();
490 return m_controlPoint->
GetId();
503 return m_controlPoint->
GetType();
529 return m_coordTypeBundle;
624 SurfacePoint::CoordUnits units = SurfacePoint::Degrees;
627 SurfacePoint::One, units);
629 SurfacePoint::Two, units);
631 SurfacePoint::Three, SurfacePoint::Kilometers);
635 QString output = QString(
"%1%2%3 of %4%5%6%7%8%9%10%11\n")
664 bool solveRadius)
const {
675 IString msg =
"Unknown surface point coordinate type enum [" +
toString(m_coordTypeBundle) +
"]." ;
703 bool errorPropagation,
704 bool solveRadius)
const {
714 double rtm = rad * 1000.;
719 double cor_lat_dd = 0.;
720 double cor_lon_dd = 0.;
721 double cor_rad_km = 0.;
722 double cor_lat_m = 0.;
723 double cor_lon_m = 0.;
724 double cor_rad_m = 0.;
730 double x = m_controlPoint->GetAdjustedSurfacePoint().GetX().
kilometers();
732 double y = m_controlPoint->GetAdjustedSurfacePoint().GetY().
kilometers();
734 double z = m_controlPoint->GetAdjustedSurfacePoint().GetZ().
kilometers();
745 cor_lat_dd = (lat - latInit);
746 cor_lat_m = cor_lat_dd *
DEG2RAD * rtm;
749 cor_lon_dd = (lon - lonInit);
753 cor_rad_km = rad - radInit;
754 cor_rad_m = cor_rad_km * 1000.;
768 latInit = lat - cor_lat_dd;
772 lonInit = lon - cor_lon_dd;
780 IString msg =
"Unknown surface point coordinate type enum [" +
781 toString(m_coordTypeBundle) +
"]." ;
789 output = QString(
" Label: %1\nStatus: %2\n Rays: %3 of %4\n")
795 QString labels =
"\n Point Initial Total Total "
796 " Final Initial Final\n"
797 "Coordinate Value Correction Correction "
798 " Value Accuracy Accuracy\n"
799 " (dd/dd/km) (dd/dd/km) (Meters) "
800 " (dd/dd/km) (Meters) (Meters)\n";
803 SurfacePoint::CoordIndex idx = SurfacePoint::One;
804 output += QString(
" LATITUDE%1%2%3%4%5%6\n")
812 idx = SurfacePoint::Two;
813 output += QString(
" LONGITUDE%1%2%3%4%5%6\n")
821 idx = SurfacePoint::Three;
822 output += QString(
" RADIUS%1%2%3%4%5%6\n\n")
846 (
bool errorPropagation)
const {
849 double X = m_controlPoint->GetAdjustedSurfacePoint().GetX().
kilometers();
850 double Y = m_controlPoint->GetAdjustedSurfacePoint().GetY().
kilometers();
851 double Z = m_controlPoint->GetAdjustedSurfacePoint().GetZ().
kilometers();
880 cor_X_m = (X - XInit);
884 cor_Y_m = (Y - YInit);
888 cor_Z_m = (Z - ZInit);
907 IString msg =
"Unknown surface point coordinate type enum [" +
908 toString(m_coordTypeBundle) +
"]." ;
916 output = QString(
" Label: %1\nStatus: %2\n Rays: %3 of %4\n")
923 "\n Point Initial Total Final "
925 " Coordinate Value Correction Value "
926 " Accuracy Accuracy\n"
927 " (km/km/km) (km) (km/km/km) "
928 " (Meters) (Meters)\n";
931 SurfacePoint::CoordIndex idx = SurfacePoint::One;
932 output += QString(
" BODY-FIXED-X%1%2%3%4%5\n")
939 idx = SurfacePoint::Two;
940 output += QString(
" BODY-FIXED-Y%1%2%3%4%5\n")
947 idx = SurfacePoint::Three;
948 output += QString(
" BODY-FIXED-Z%1%2%3%4%5\n\n")
974 output = QString(
"%1").arg(
"Null", fieldWidth) :
975 output = QString(
"%1").arg(value, fieldWidth,
'f', precision);
994 int fieldWidth,
int precision,
995 bool solveRadius)
const {
996 QString aprioriSigmaStr;
998 if (pointType ==
"CONSTRAINED" || !solveRadius) {
1003 aprioriSigmaStr = QString(
"%1").arg(pointType, fieldWidth);
1006 aprioriSigmaStr = QString(
"%1").arg(sigma, fieldWidth,
'f', precision);
1008 return aprioriSigmaStr;
1025 bool solveRadius)
const {
1044 int fieldWidth,
int precision,
1045 bool errorPropagation)
const {
1046 QString adjustedSigmaStr;
1048 if (!errorPropagation) {
1049 adjustedSigmaStr = QString(
"%1").arg(
"N/A",fieldWidth);
1056 adjustedSigmaStr = QString(
"%1").arg(
"N/A",fieldWidth);
1059 adjustedSigmaStr = QString(
"%1").arg(sigma, fieldWidth,
'f', precision);
1063 return adjustedSigmaStr;
1080 int fieldWidth,
int precision,
1081 bool errorPropagation)
const {
1105 pointLat +=
RAD2DEG * latCorrection;
1106 pointLon +=
RAD2DEG * lonCorrection;
1109 if (pointLat < -90.0) {
1110 pointLat = -180.0 - pointLat;
1111 pointLon = pointLon + 180.0;
1113 if (pointLat > 90.0) {
1114 pointLat = 180.0 - pointLat;
1115 pointLon = pointLon + 180.0;
1117 while (pointLon > 360.0) {
1118 pointLon = pointLon - 360.0;
1120 while (pointLon < 0.0) {
1121 pointLon = pointLon + 360.0;
1125 pointRad += 1000. * radCorrection;
1130 if (target && (target->solveMeanRadius() || target->solveTriaxialRadii()) ) {
1131 if (target->solveMeanRadius()) {
1134 target->meanRadius());
1136 else if (target->solveTriaxialRadii()) {
1161 double pointX = surfacepoint.GetX().
meters();
1162 double pointY = surfacepoint.GetY().
meters();
1163 double pointZ = surfacepoint.GetZ().
meters();
1171 pointX += 1000. * XCorrection;
1172 pointY += 1000. * YCorrection;
1173 pointZ += 1000. * ZCorrection;
void setAdjustedSurfacePoint(SurfacePoint surfacePoint)
Sets the adjusted surface point for this BundleControlPoint.
~BundleControlPoint()
Destructor for BundleControlPoint.
double kilometers() const
Get the distance in kilometers.
void applyParameterCorrections(LinearAlgebra::Vector imageSolution, SparseBlockMatrix &sparseNormals, const BundleTargetBodyQsp target)
Apply the parameter corrections to the bundle control point.
@ Degrees
Degrees are generally considered more human readable, 0-360 is one circle, however most math does not...
QString formatAdjustedSigmaString(SurfacePoint::CoordIndex, int fieldWidth, int precision, bool errorPropagation) const
Formats the adjusted sigma value indicated by the given type code.
double MetersToLatitude(double latLength)
This method returns an angular measure of a distance in the direction of and relative to the latitude...
int numberOfRejectedMeasures() const
Accesses the number of rejected measures for this BundleControlPoint.
void updateAdjustedSurfacePointRectangularly()
pointer to the control point object this represents
static QString PointTypeToString(PointType type)
Obtain a string representation of a given PointType.
@ Meters
The distance is being specified in meters.
SurfacePoint adjustedSurfacePoint() const
Accesses the adjusted SurfacePoint associated with this BundleControlPoint.
void ZeroNumberOfRejectedMeasures()
Initialize the number of rejected measures to 0.
bool IsCoord3Constrained()
Return bool indicating if 3rd coordinate is Constrained or not.
ControlPoint::PointType type() const
Accesses BundleControlPoint's type.
const ControlMeasure * GetMeasure(QString serialNumber) const
Get a control measure based on its cube's serial number.
This class is designed to encapsulate the concept of a Latitude.
SurfacePoint::CoordinateType m_coordTypeReports
BundleControlPoint coordinate type.
boost::numeric::ublas::bounded_vector< double, 3 > & weights()
Accesses the 3 dimensional ordered vector of weight values associated with coordinate1,...
SparseBlockRowMatrix m_cholmodQMatrix
The CholMod matrix associated with this point.
const double DEG2RAD
Multiplier for converting from degrees to radians.
double GetResidualRms() const
Get rms of residuals.
void setRejected(bool reject)
Sets this BundleControlPoint to rejected or not rejected.
Status SetAdjustedSurfacePoint(SurfacePoint newSurfacePoint)
Set or update the surface point relating to this control point.
void SetRectangularCoordinates(const Displacement &x, const Displacement &y, const Displacement &z)
Set surface point in rectangular coordinates.
void SetNumberOfRejectedMeasures(int numRejected)
Set (update) the number of rejected measures for the control point.
void setWeights(const BundleSettingsQsp settings)
Sets the weights using the given BundleSettings QSharedPointer and a conversion value for meters to r...
boost::numeric::ublas::bounded_vector< double, 3 > m_weights
weights for point parameters
bool isRejected() const
Method used to determine whether this control point is rejected.
PointType
These are the valid 'types' of point.
Latitude GetLatitude() const
Return the body-fixed latitude for the surface point.
This class holds information about a control point that BundleAdjust needs to run correctly.
int GetNumberOfRejectedMeasures() const
Get the number of rejected measures on the control point.
QString GetId() const
Return the Id of the control point.
boost::numeric::ublas::bounded_vector< double, 3 > & corrections()
Accesses the 3 dimensional ordered vector of correction values associated with coord1,...
QString id() const
Accesses the Point ID associated with this BundleControlPoint.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
QString formatBundleLatitudinalOutputDetailString(bool errorPropagation, bool solveRadius=false) const
Formats a detailed output string table for this Latitudinal BundleControlPoint.
bool IsSpecial(const double d)
Returns if the input pixel is special.
double residualRms() const
Gets the root-mean-square (rms) of the BundleControlPoint's residuals.
boost::numeric::ublas::bounded_vector< double, 3 > & aprioriSigmas()
Accesses the 3 dimensional ordered vector of apriori sigmas (apriori coordinate1, apriori coordinate2...
double GetCoord(CoordinateType type, CoordIndex index, CoordUnits units)
This method returns a coordinate of a SurfacePoint.
Distance measurement, usually in meters.
This class is designed to encapsulate the concept of a Longitude.
QString formatBundleRectangularOutputDetailString(bool errorPropagation) const
Formats a detailed output string table for this Rectangular BundleControlPoint.
QSharedPointer< BundleMeasure > BundleMeasureQsp
Definition for BundleMeasureQsp, a shared pointer to a BundleMeasure.
double LongitudeToMeters(double longitude) const
This method returns a length in meters version of a delta longitude angle in radians relative to the ...
void setSigmaWeightFromGlobals(double gSigma, int index)
Sets the member sigmas and weights from a global sigma.
@ Kilometers
The distance is being specified in kilometers.
Displacement is a signed length, usually in meters.
BundleControlPoint(BundleSettingsQsp bundleSettings, ControlPoint *point)
Constructs a BundleControlPoint object from a ControlPoint.
SurfacePoint::CoordinateType coordTypeReports() const
Accesses BundleControlPoint's coordinate type for reports.
@ Fixed
A Fixed point is a Control Point whose lat/lon is well established and should not be changed.
@ Meters
The distance is being specified in meters.
bool IsCoord2Constrained()
Return bool indicating if 2nd coordinate is Constrained or not.
double meters() const
Get the displacement in meters.
@ Rectangular
Body-fixed rectangular x/y/z coordinates.
boost::numeric::ublas::bounded_vector< double, 3 > & nicVector()
Accesses the 3 dimensional ordered NIC vector.
boost::numeric::ublas::vector< double > Vector
Definition for an Isis::LinearAlgebra::Vector of doubles.
CoordinateType
Defines the coordinate typ, units, and coordinate index for some of the output methods.
QString formatCoordAprioriSigmaString(SurfacePoint::CoordIndex index, int fieldWidth, int precision, bool solveRadius=false) const
Formats the apriori coordinate 1 (latitude or X) sigma value.
void computeResiduals()
Computes the residuals for this BundleControlPoint.
boost::numeric::ublas::bounded_vector< double, 3 > m_adjustedSigmas
adjusted sigmas for point parameters
double LatitudeToMeters(double latitude) const
This method returns a Displacement of an Angle relative to the current SurfacePoint latitude.
@ Constrained
A Constrained point is a Control Point whose lat/lon/radius is somewhat established and should not be...
boost::numeric::ublas::bounded_vector< double, 3 > m_aprioriSigmas
apriori sigmas for point parameters
double MetersToLongitude(double lonLength)
This method returns an angular measure in radians of a distance in the direction of and relative to t...
QString formatValue(double value, int fieldWidth, int precision) const
Formats the given double precision value using the specified field width and precision.
void zeroNumberOfRejectedMeasures()
Resets the number of rejected measures for this BundleControlPoint to zero.
QString formatBundleOutputSummaryString(bool errorPropagation) const
Formats an output summary string for this BundleControlPoint.
A container class for a ControlMeasure.
ControlPoint * rawControlPoint() const
Accessor for the raw ControlPoint object used for this BundleControlPoint.
Longitude GetLongitude() const
Return the body-fixed longitude for the surface point.
const double Null
Value for an Isis Null pixel.
double kilometers() const
Get the displacement in kilometers.
BundleMeasureQsp addMeasure(ControlMeasure *controlMeasure)
Creates a BundleMeasure from the given ControlMeasure and appends it to this BundleControlPoint's mea...
void setNumberOfRejectedMeasures(int numRejected)
Sets the number of rejected measures for this BundleControlPoint.
int numberOfMeasures() const
Accesses number of measures associated with this BundleControlPoint.
void updateAdjustedSurfacePointLatitudinally(const BundleTargetBodyQsp target)
Apply the parameter corrections to the bundle control point latitudinally.
Distance GetLocalRadius() const
Return the radius of the surface point.
@ Programmer
This error is for when a programmer made an API call that was illegal.
bool IsCoord1Constrained()
Return bool indicating if 1st coordinate is Constrained or not.
void SetSphericalCoordinates(const Latitude &lat, const Longitude &lon, const Distance &radius)
Update spherical coordinates (lat/lon/radius)
boost::numeric::ublas::bounded_vector< double, 3 > & adjustedSigmas()
Accesses the 3 dimensional ordered vector of adjusted sigmas (adjusted coordinate1,...
QString formatCoordAdjustedSigmaString(SurfacePoint::CoordIndex, int fieldWidth, int precision, bool errorPropagation) const
Formats the adjusted coordinate sigma value.
QString formatAprioriSigmaString(SurfacePoint::CoordIndex index, int fieldWidth, int precision, bool solveRadius=false) const
Formats the apriori sigma value indicated by the given type code.
void productAlphaAV(double alpha, SparseBlockMatrix &sparseNormals, LinearAlgebra::Vector &v1)
Perform the matrix multiplication v2 = alpha ( Q x v1 ).
@ Free
A Free point is a Control Point that identifies common measurements between two or more cubes.
double meters() const
Get the distance in meters.
double degrees() const
Get the angle in units of Degrees.
PointType GetType() const
Status SetRejected(bool rejected)
Set the jigsawRejected state.
Distance GetSigmaDistance(CoordinateType type, CoordIndex index)
This method returns a sigma of a SurfacePoint coordinate as a Distance.
boost::numeric::ublas::bounded_vector< double, 3 > m_corrections
corrections to point parameters
QString formatBundleOutputDetailString(bool errorPropagation, bool solveRadius=false) const
Formats a detailed output string table for this BundleControlPoint.
@ Latitudinal
Planetocentric latitudinal (lat/lon/rad) coordinates.
SparseBlockRowMatrix & cholmodQMatrix()
Accesses the CholMod matrix associated with this BundleControlPoint.
Adds specific functionality to C++ strings.
SurfacePoint::CoordinateType coordTypeBundle() const
Accesses BundleControlPoint's control point coordinate type for the bundle adjustment.
boost::numeric::ublas::bounded_vector< double, 3 > m_nicVector
array of NICs (see Brown, 1976)
double GetWeight(CoordinateType type, CoordIndex index)
This method returns the weight of a SurfacePoint coordinate Note: At this time a units argument is no...
const double RAD2DEG
Multiplier for converting from radians to degrees.
@ Kilometers
The distance is being specified in kilometers.
This class defines a body-fixed surface point.
This is free and unencumbered software released into the public domain.
Status ComputeResiduals()
This method computes the BundleAdjust residuals for a point.
void copy(const BundleControlPoint &src)
Copies given BundleControlPoint to this BundleControlPoint.