File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
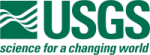 |
Isis 3 Programmer Reference
|
7 #include "EllipsoidShape.h"
17 #include "IException.h"
20 #include "Longitude.h"
21 #include "NaifStatus.h"
22 #include "ShapeModel.h"
23 #include "SurfacePoint.h"
53 std::vector<double> lookDirection) {
111 IString msg =
"A valid intersection must be defined before computing the surface normal";
123 double a = radii[0].kilometers();
124 double b = radii[1].kilometers();
125 double c = radii[2].kilometers();
127 vector<double>
normal(3,0.);
129 surfnm_c(a, b, c, pB, (SpiceDouble *) &
normal[0]);
148 double a = radii[0].kilometers();
149 double b = radii[1].kilometers();
150 double c = radii[2].kilometers();
155 double xyradius = a * b / sqrt(pow(b * cos(rlon), 2) +
156 pow(a * sin(rlon), 2));
157 const double &radius = xyradius * c / sqrt(pow(c * cos(rlat), 2) +
158 pow(xyradius * sin(rlat), 2));
Distance localRadius(const Latitude &lat, const Longitude &lon)
Get the local radius for a point on the surface.
This class is designed to encapsulate the concept of a Latitude.
SurfacePoint * surfaceIntersection() const
Returns the surface intersection for this ShapeModel.
std::vector< Distance > targetRadii() const
Returns the radii of the body in km.
virtual void calculateDefaultNormal()
Calculate the default normal of the current intersection point.
bool intersectSurface(std::vector< double > observerPos, std::vector< double > lookDirection)
Intersect the shape model.
void calculateSurfaceNormal()
Calculate the surface normal of the current intersection point.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
void setNormal(const std::vector< double >)
Sets the normal for the currect intersection point.
Distance measurement, usually in meters.
This class is designed to encapsulate the concept of a Longitude.
@ Kilometers
The distance is being specified in kilometers.
void setName(QString name)
Sets the shape name.
EllipsoidShape()
Initialize the EllipsoidShape.
bool intersectEllipsoid(const std::vector< double > observerPosRelativeToTarget, const std::vector< double > &observerLookVectorToTarget)
Finds the intersection point on the ellipsoid model using the given position of the observer (spacecr...
void calculateLocalNormal(QVector< double * > cornerNeighborPoints)
Calculate the local surface normal of the current intersection point.
void setHasNormal(bool status)
Sets the flag to indicate whether this ShapeModel has a surface normal.
double kilometers() const
Get the displacement in kilometers.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
Define shapes and provide utilities for Isis targets.
bool hasIntersection()
Returns intersection status.
Adds specific functionality to C++ strings.
This is free and unencumbered software released into the public domain.
This class is used to create and store valid Isis targets.
bool isDEM() const
Indicates that this shape model is not from a DEM.
This is free and unencumbered software released into the public domain.
std::vector< double > normal()
Returns the local surface normal at the current intersection point.
double radians() const
Convert an angle to a double.