File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
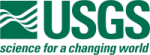 |
Isis 3 Programmer Reference
|
14 #include "MdisCamera.h"
15 #include "TaylorCameraDistortionMap.h"
17 #include "CameraDetectorMap.h"
18 #include "CameraDistortionMap.h"
19 #include "CameraFocalPlaneMap.h"
20 #include "CameraGroundMap.h"
21 #include "CameraSkyMap.h"
22 #include "IException.h"
25 #include "NaifStatus.h"
59 const int MdisWac(-236800);
60 const int MdisNac(-236820);
72 msg +=
" is not a supported instrument kernel code for Messenger.";
84 #if defined(MDIS_SUBFRAMES_UNSUPPORTED)
85 int subFrameMode = inst[
"SubFrameMode"];
86 if(subFrameMode != 0) {
87 string msg =
"Subframe imaging mode is not supported!";
88 throw iException::Message(iException::User, msg, _FILEINFO_);
95 #if defined(MDIS_JAILBARS_UNSUPPORTED)
96 int jailBars = inst[
"JailBars"];
98 string msg =
"Jail bar observations are not currently supported!";
99 throw iException::Message(iException::Programmer, msg, _FILEINFO_);
108 filterNumber = bandBin[
"Number"];
114 QString filterCode =
toString(fnCode);
118 ikernKey =
"INS" + ikCode +
"_REFERENCE_FRAME";
121 ikernKey =
"INS" + filterCode +
"_FRAME";
142 QString stime = inst[
"SpacecraftClockCount"];
155 ikernKey =
"INS" + ikCode +
"_BORESIGHT_SAMPLE";
156 double sampleBoreSight =
getDouble(ikernKey);
158 ikernKey =
"INS" + ikCode +
"_BORESIGHT_LINE";
159 double lineBoreSight =
getDouble(ikernKey);
174 int fpuBinMode = inst[
"FpuBinningMode"];
175 int pixelBinMode = inst[
"PixelBinningMode"];
177 int summing = ((pixelBinMode == 0) ? 1 : pixelBinMode);
180 if(fpuBinMode == 1) {
181 #if defined(USE_FPU_BINNING_OFFSETS)
182 ikernKey =
"INS" + ikCode +
"_FPUBIN_START_SAMPLE";
183 double fpuStartingSample =
getDouble(ikernKey);
186 ikernKey =
"INS" + ikCode +
"_FPUBIN_START_LINE";
187 double fpuStartingLine =
getDouble(ikernKey);
213 string msg =
"New MDIS NAC/WAC distortion models will invalidate previous "
214 "SPICE - you may need to rerun spiceinit to get new kernels";
258 double exposureDuration) {
295 double focalLength(0.0);
296 QString tdflKey(
"TempDependentFocalLength");
302 if (my_tdfl.isValid()) {
310 focalLength =
getDouble(
"INS" + filterCode +
"_FOCAL_LENGTH");
313 bool tdfl_disabled(
false);
314 #ifndef DISABLE_TDFL_DISABLING
317 tdfl_disabled = (
"TRUE" == tdfl_state.
UpCase() );
320 tdfl_disabled =
false;
326 if ( !tdfl_disabled ) {
331 double fpTemp = inst[
"FocalPlaneTemperature"];
333 QString fptCoeffs =
"INS" + filterCode +
"_FL_TEMP_COEFFS";
335 for (
int i = 0 ; i < 6 ; i++) {
336 fl +=
getDouble(fptCoeffs, i) * pow(fpTemp, (
double) i);
346 "Failed to compute temperature-dependent focal length",
351 return (focalLength);
MESSENGER MDIS NAC and WAC Camera Model.
double ToDouble() const
Returns the floating point value the IString represents.
void SetDetectorOrigin(const double sample, const double line)
Set the detector origin.
virtual double exposureDuration() const
Return the exposure duration for the pixel that the camera is set to.
iTime time() const
Returns the ephemeris time in seconds which was used to obtain the spacecraft and sun positions.
Distort/undistort focal plane coordinates.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
Convert between parent image coordinates and detector coordinates.
SpiceInt naifIkCode() const
This returns the NAIF IK code to use when reading from instrument kernels.
void SetDistortion(const int naifIkCode)
Load distortion coefficients.
QString getString(const QString &key, int index=0)
This returns a value from the NAIF text pool.
Parse and return pieces of a time string.
QString m_instrumentNameLong
Full instrument name.
virtual iTime getClockTime(QString clockValue, int sclkCode=-1, bool clockTicks=false)
This converts the spacecraft clock ticks value (clockValue) to an iTime.
void SetDetectorSampleSumming(const double summing)
Set sample summing mode.
Container for cube-like labels.
virtual std::pair< iTime, iTime > ShutterOpenCloseTimes(double time, double exposureDuration)=0
Returns the shutter open and close times.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
Generic class for Framing Cameras.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
Convert between undistorted focal plane and ground coordinates.
void SetDetectorLineSumming(const double summing)
Set line summing mode.
void SetStartingDetectorLine(const double line)
Set the starting detector line.
@ Traverse
Search child objects.
IString UpCase()
Converst any lower case characters in the object IString with uppercase characters.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
void SetPixelPitch()
Reads the Pixel Pitch from the instrument kernel.
Contains multiple PvlContainers.
@ SpiceStringType
SpiceString type.
IO Handler for Isis Cubes.
QString m_spacecraftNameLong
Full spacecraft name.
double computeFocalLength(const QString &filterCode, Pvl &label)
Computes temperature-dependent focal length.
virtual std::pair< iTime, iTime > ShutterOpenCloseTimes(double time, double exposureDuration)
Returns the shutter open and close times.
void SetStartingDetectorSample(const double sample)
Set the starting detector sample.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
void LoadCache()
This loads the spice cache big enough for this image.
QString m_instrumentNameShort
Shortened instrument name.
Convert between distorted focal plane and detector coordinates.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
Convert between undistorted focal plane and ra/dec coordinates.
Adds specific functionality to C++ strings.
SpiceDouble getDouble(const QString &key, int index=0)
This returns a value from the NAIF text pool.
QString m_spacecraftNameShort
Shortened spacecraft name.
void SetFocalLength()
Reads the focal length from the instrument kernel.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....