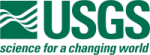 |
Isis 3 Programmer Reference
|
10 #include "RosettaOsirisCamera.h"
16 #include "CameraDetectorMap.h"
17 #include "CameraFocalPlaneMap.h"
18 #include "CameraGroundMap.h"
19 #include "CameraSkyMap.h"
23 #include "NaifStatus.h"
24 #include "Preference.h"
58 QString fl =
"INS" + ikCode +
"_FOCAL_LENGTH";
64 QString pp =
"INS" + ikCode +
"_PIXEL_SIZE";
81 double pixelAveragingWidth=(double) inst[
"PixelAveragingWidth"];
82 double pixelAveragingHeight=(double) inst[
"PixelAveragingHeight"];
93 QString clockStartCount = inst[
"SpacecraftClockStartCount"];
97 double exposureTime = (double) inst[
"ExposureDuration"];
101 QString filterNumber = BandBin[
"FilterNumber"];
107 double referenceSample =
Spice::getDouble(
"INS" + ikCode +
"_BORESIGHT",0) + 1.0;
108 double referenceLine =
Spice::getDouble(
"INS" + ikCode +
"_BORESIGHT",1) + 1.0;
114 distortionMap->
setBoresight(referenceSample, referenceLine);
116 iTime centerTime = start + (exposureTime / 2.0);
146 double exposureDuration) {
165 for (
int i = 0; i < 4; i++) {
166 for (
int j = 0; j < 4; j++) {
167 toUnDistX(i, j) =
Spice::getDouble(
"INS" + ikCode +
"_TO_UNDISTORTED_X", 4 * i + j);
168 toUnDistY(i, j) =
Spice::getDouble(
"INS" + ikCode +
"_TO_UNDISTORTED_Y", 4 * i + j);
void SetDetectorOrigin(const double sample, const double line)
Set the detector origin.
void setUnDistortedXMatrix(LinearAlgebra::Matrix xMat)
Set the matrix for converting from distorted to undistorted samples.
virtual double exposureDuration() const
Return the exposure duration for the pixel that the camera is set to.
iTime time() const
Returns the ephemeris time in seconds which was used to obtain the spacecraft and sun positions.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
Convert between parent image coordinates and detector coordinates.
SpiceInt naifIkCode() const
This returns the NAIF IK code to use when reading from instrument kernels.
void setBoresight(double sample, double line)
Set the boresight location for converting from focal plane coordinates to pixel coordinates.
Parse and return pieces of a time string.
QString m_instrumentNameLong
Full instrument name.
virtual iTime getClockTime(QString clockValue, int sclkCode=-1, bool clockTicks=false)
This converts the spacecraft clock ticks value (clockValue) to an iTime.
virtual std::pair< iTime, iTime > ShutterOpenCloseTimes(double time, double exposureDuration)
Reimplemented from FrameCamera.
void SetDetectorSampleSumming(const double summing)
Set sample summing mode.
void initDistortion(QString ikCode, RosettaOsirisCameraDistortionMap *distortionMap)
Initialize the distortion map using the paramters from the NAIF SPICE kernels.
static Matrix zeroMatrix(int rows, int columns)
Returns a matrix with given dimensions that is filled with zeroes.
Container for cube-like labels.
virtual std::pair< iTime, iTime > ShutterOpenCloseTimes(double time, double exposureDuration)=0
Returns the shutter open and close times.
void setPixelPitch(double pitch)
Set the pixel pitch for converting from focal plane coordinates to pixel coordinates.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
boost::numeric::ublas::matrix< double > Matrix
Definition for an Isis::LinearAlgebra::Matrix of doubles.
Generic class for Framing Cameras.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
Convert between undistorted focal plane and ground coordinates.
void SetDetectorLineSumming(const double summing)
Set line summing mode.
void SetStartingDetectorLine(const double line)
Set the starting detector line.
@ Traverse
Search child objects.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
void SetPixelPitch()
Reads the Pixel Pitch from the instrument kernel.
Contains multiple PvlContainers.
IO Handler for Isis Cubes.
QString m_spacecraftNameLong
Full spacecraft name.
void SetStartingDetectorSample(const double sample)
Set the starting detector sample.
This is the camera model for the Osiris NAC Framing Camera.
Namespace for the standard library.
void LoadCache()
This loads the spice cache big enough for this image.
QString m_instrumentNameShort
Shortened instrument name.
Convert between distorted focal plane and detector coordinates.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
Convert between undistorted focal plane and ra/dec coordinates.
void setUnDistortedYMatrix(LinearAlgebra::Matrix yMat)
Set the matrix for converting from distorted to undistorted lines.
SpiceDouble getDouble(const QString &key, int index=0)
This returns a value from the NAIF text pool.
QString m_spacecraftNameShort
Shortened spacecraft name.
void SetFocalLength()
Reads the focal length from the instrument kernel.
This is free and unencumbered software released into the public domain.
Distortion map for converting between undistorted focal plane and distorted focal plane coordinates f...