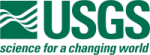 |
Isis 3 Programmer Reference
|
1 #include "StretchTool.h"
10 #include <QMessageBox>
12 #include <QPushButton>
13 #include <QSizePolicy>
14 #include <QStackedWidget>
16 #include <QToolButton>
18 #include <QInputDialog>
20 #include "AdvancedStretchDialog.h"
23 #include "CubeViewport.h"
24 #include "Histogram.h"
25 #include "IException.h"
27 #include "MainWindow.h"
28 #include "MdiCubeViewport.h"
29 #include "RubberBandTool.h"
30 #include "Statistics.h"
33 #include "ViewportBuffer.h"
34 #include "ViewportMainWindow.h"
35 #include "Workspace.h"
37 #include "CubeStretch.h"
67 QPushButton *hiddenButton =
new QPushButton();
68 hiddenButton->setVisible(
false);
69 hiddenButton->setDefault(
true);
84 if (parentMainWindow) {
85 connect(
this, SIGNAL(
warningSignal(std::string &,
const std::string)),
86 parentMainWindow, SLOT(displayWarning(std::string &,
const std::string &)));
113 action->setIcon(QPixmap(
toolIconDir() +
"/stretch_global.png"));
114 action->setToolTip(
"Stretch (S)");
115 action->setShortcut(Qt::Key_S);
117 "<b>Function:</b> Change the stretch range of the cube.\
118 <p><b>Shortcut:</b> S</p> ";
119 action->setWhatsThis(text);
148 QToolButton *butt =
new QToolButton(hbox);
149 butt->setAutoRaise(
true);
150 butt->setIconSize(QSize(22, 22));
151 butt->setIcon(QPixmap(
toolIconDir() +
"/regional_stretch-2.png"));
152 butt->setToolTip(
"Stretch");
154 "<b>Function:</b> Automatically compute min/max stretch using viewed \
155 pixels in the band(s) of the active viewport. That is, only pixels \
156 that are visible in the viewport are used. \
157 If the viewport is in RGB color all three bands will be stretched. \
158 <p><b>Shortcut:</b> Ctrl+R</p> \
159 <p><b>Mouse:</b> Left click \
160 <p><b>Hint:</b> Left click and drag for a local stretch. Uses only \
161 pixels in the red marquee</p>";
162 butt->setWhatsThis(text);
174 "<b>Function:</b> Selecting the color will allow the appropriate \
175 min/max to be seen and/or edited in text fields to the right.";
186 QDoubleValidator *dval =
new QDoubleValidator(hbox);
191 "<b>Function:</b> Shows the current minimum pixel value. Pixel values \
192 below minimum are shown as black. Pixel values above the maximum \
193 are shown as white or the highest intensity of red/green/blue \
194 if in color. Pixel values between the minimum and maximum are stretched \
195 linearly between black and white (or color component). \
196 <p><b>Hint:</b> You can manually edit the minimum but it must be \
197 less than the maximum.";
207 "<b>Function:</b> Shows the current maximum pixel value. Pixel values \
208 below minimum are shown as black. Pixel values above the maximum \
209 are shown as white or the highest intensity of red/green/blue \
210 if in color. Pixel values between the minimum and maximum are stretched \
211 linearly between black and white (or color component). \
212 <p><b>Hint:</b> You can manually edit the maximum but it must be \
213 greater than the minimum";
227 copyAll->setIcon(QPixmap(
toolIconDir() +
"/copy_stretch.png"));
228 copyAll->setText(
"to All Viewports");
231 copyMenu->addAction(copyAll);
238 m_copyButton->setPopupMode(QToolButton::MenuButtonPopup);
243 "<b>Function:</b> Copy the current stretch to all the \
244 active viewports. Or use the drop down menu to copy the current stretch \
245 to all the bands in the active viewport. \
246 <p><b>Hint:</b> Can reset the stretch to an automaticaly computed \
247 stretch by using the 'Reset' stretch button option. </p>";
251 currentView->setText(
"Active Viewport");
252 currentView->setIcon(QPixmap(
toolIconDir() +
"/global_stretch.png"));
253 globalMenu->addAction(currentView);
254 connect(currentView, SIGNAL(triggered(
bool)),
this, SLOT(
stretchGlobal()));
257 globalAll->setText(
"All Viewports");
258 globalMenu->addAction(globalAll);
262 globalBands->setText(
"All Bands");
263 globalMenu->addAction(globalBands);
274 "<b>Function:</b> Reset the stretch to be automatically computed "
275 "using the statisics from the entire image. Use the drop down menu "
276 "to reset the stretch for all the bands in the active viewport or "
277 "to reset the stretch for all the viewports.";
280 QPushButton *advancedButton =
new QPushButton(
"Advanced");
285 "<b>Function:</b> While this button is pressed down, the visible stretch "
286 "will be the automatically computed stretch using the statisics from the "
287 "entire image. The original stretch is restored once you let up on this "
294 QPushButton *saveToCubeButton =
new QPushButton(
"Save");
295 connect(saveToCubeButton, SIGNAL(clicked(
bool)),
this, SLOT(
saveStretchToCube()));
297 QPushButton *deleteFromCubeButton =
new QPushButton(
"Delete");
298 connect(deleteFromCubeButton, SIGNAL(clicked(
bool)),
this, SLOT(
deleteFromCube()));
300 QPushButton *loadStretchButton =
new QPushButton(
"Restore");
303 QHBoxLayout *layout =
new QHBoxLayout(hbox);
304 layout->setMargin(0);
311 layout->addWidget(advancedButton);
316 layout->addWidget(saveToCubeButton);
317 layout->addWidget(deleteFromCubeButton);
318 layout->addWidget(loadStretchButton);
320 layout->addStretch();
321 hbox->setLayout(layout);
398 bluStretch, bluHist);
417 for (objIter=lab->
beginObject(); objIter<lab->endObject(); objIter++) {
418 if (objIter->name() ==
"Stretch") {
419 PvlKeyword tempKeyword = objIter->findKeyword(
"Name");
420 int bandNumber = int(objIter->findKeyword(
"BandNumber"));
421 if (cvp->
grayBand() == bandNumber) {
422 QString tempName = tempKeyword[0];
423 namelist.append(tempName);
429 int redBandNumber = cvp->
redBand();
431 int blueBandNumber = cvp->
blueBand();
435 for (objIter=lab->
beginObject(); objIter<lab->endObject(); objIter++) {
436 if (objIter->name() ==
"Stretch") {
437 PvlKeyword tempKeyword = objIter->findKeyword(
"Name");
438 int bandNumber = int(objIter->findKeyword(
"BandNumber"));
439 if (bandNumber == redBandNumber || bandNumber == greenBandNumber
440 || bandNumber == blueBandNumber) {
441 QString tempName = tempKeyword[0];
442 if (tempNameMap.contains(tempName)) {
443 tempNameMap[tempName].append(bandNumber);
447 tempNameMap[tempName] = {bandNumber};
453 while (i != tempNameMap.constEnd()) {
454 if (i.value().contains(redBandNumber) && i.value().contains(greenBandNumber) &&
455 i.value().contains(blueBandNumber) ){
456 namelist.append(i.key());
465 if (namelist.size() >=1) {
466 stretchName = QInputDialog::getItem((
QWidget *)parent(), tr(
"Load Stretch"),
467 tr(
"Name of Stretch to Load:"), namelist, 0,
471 QMessageBox::information((
QWidget *)parent(),
"Information",
472 "There are no saved stretches to restore.");
488 std::vector<PvlKeyword> keywordValueRed;
489 keywordValueRed.push_back(
PvlKeyword(
"BandNumber", QString::number(cvp->
redBand())));
491 std::vector<PvlKeyword> keywordValueGreen;
494 std::vector<PvlKeyword> keywordValueBlue;
495 keywordValueBlue.push_back(
PvlKeyword(
"BandNumber", QString::number(cvp->
blueBand())));
535 for (objIter=lab->
beginObject(); objIter<lab->endObject(); objIter++) {
536 if (objIter->name() ==
"Stretch") {
537 PvlKeyword tempKeyword = objIter->findKeyword(
"Name");
538 int bandNumber = int(objIter->findKeyword(
"BandNumber"));
539 if (cvp->
grayBand() == bandNumber) {
540 QString tempName = tempKeyword[0];
541 namelist.append(tempName);
549 if (namelist.size() >= 1) {
550 toDelete = QInputDialog::getItem((
QWidget *)parent(), tr(
"Delete Stretch"),
551 tr(
"Name of Stretch to Delete:"), namelist, 0,
555 QMessageBox::information((
QWidget *)parent(),
"Information",
556 "There are no saved stretches to delete.");
566 QMessageBox::information((
QWidget *)parent(),
"Error",
567 "Cannot open cube read/write to delete stretch");
572 bool cubeDeleted = icube->
deleteBlob(toDelete,
"Stretch");
576 msgBox.setText(
"Stretch Could Not Be Deleted!");
577 msgBox.setInformativeText(
"A stretch with name: \"" + toDelete +
578 "\" Could not be found, so there was nothing to delete from the Cube.");
579 msgBox.setStandardButtons(QMessageBox::Ok);
580 msgBox.setIcon(QMessageBox::Critical);
601 for (objIter=lab->
beginObject(); objIter<lab->endObject(); objIter++) {
602 if (objIter->name() ==
"Stretch") {
603 PvlKeyword tempKeyword = objIter->findKeyword(
"Name");
604 QString tempName = tempKeyword[0];
605 namelist.append(tempName);
639 if (((redBand == greenBand) && !(redStretch == greenStretch)) ||
640 ((redBand == blueBand) && !(redBand == blueBand)) ||
641 ((greenBand == blueBand) && !(greenBand == blueBand))) {
642 QMessageBox::information((
QWidget *)parent(),
"Error",
"Sorry, cannot save RGB stretches which include the same band multiple times, but have different stretches for each");
649 tr(
"Enter a name to save the stretch as:"), QLineEdit::Normal,
654 if (namelist.contains(text)) {
656 msgBox.setText(tr(
"Stretch Name Already Exists!"));
657 msgBox.setInformativeText(
"A stretch pair with name: \"" + text +
"\" already exists and "
658 "the existing saved data will be overwritten. Are you sure you "
660 msgBox.setStandardButtons(QMessageBox::Save | QMessageBox::Cancel);
661 msgBox.setIcon(QMessageBox::Warning);
662 msgBox.setDefaultButton(QMessageBox::Cancel);
663 int ret = msgBox.exec();
666 case QMessageBox::Save:
668 case QMessageBox::Cancel:
686 QMessageBox::information((
QWidget *)parent(),
"Error",
"Cannot open cube read/write to save stretch");
707 icube->
write(stretch);
725 icube->
write(stretchBlob,
false);
729 stretchBlob = greenStretch.
toBlob();
730 icube->
write(stretchBlob,
false);
734 stretchBlob = blueStretch.
toBlob();
735 icube->
write(stretchBlob,
false);
779 bluStretch, bluHist);
811 if(cvp && cvp->
isGray()) {
852 QRect rect(0, 0, cvp->viewport()->width(), cvp->viewport()->height());
854 if(bandId == (
int)
Gray) {
867 if(bandId == (
int)
Red || bandId == (
int)
All) {
878 if(bandId == (
int)
Green || bandId == (
int)
All) {
889 if(bandId == (
int)
Blue || bandId == (
int)
All) {
912 if(cvp == NULL)
return;
940 double min = 0, max = 0;
945 min = stretch.
Input(0);
957 min = rstretch.
Input(0);
961 min = gstretch.
Input(0);
965 min = bstretch.
Input(0);
996 if(cvp == NULL)
return;
1036 if(cvp == NULL)
return;
1070 redStretch.
AddPair(max, 255.0);
1074 greenStretch.
AddPair(min, 0.0);
1075 greenStretch.
AddPair(max, 255.0);
1079 blueStretch.
AddPair(min, 0.0);
1080 blueStretch.
AddPair(max, 255.0);
1113 if(cvp == NULL)
return;
1124 if(cvp == NULL)
return;
1160 if(cvp == NULL)
return;
1171 QRect rect(0, 0, cvp->viewport()->width(), cvp->viewport()->height());
1176 QString message =
"Cannot stretch while the cube is still loading";
1177 QMessageBox::warning((
QWidget *)parent(),
"Warning", message);
1191 if(cvp == NULL)
return;
1192 if(!rubberBandTool()->isValid())
return;
1194 QRect rubberBandRect = rubberBandTool()->
rectangle();
1196 if(rubberBandRect.width() == 0 || rubberBandRect.height() == 0)
return;
1242 "Unknown stretch band",
1260 if(cvp == NULL)
return;
1266 if(s == Qt::RightButton) {
1283 rubberBandTool()->
enable(RubberBandTool::RectangleMode);
1295 if(cvp == NULL)
return;
1347 if(thisViewport == NULL)
return;
1403 if(stretch.
Pairs() == 0) {
1404 stretch.
AddPair(-DBL_MAX, 0.0);
1405 stretch.
AddPair(DBL_MAX, 255.0);
1426 else if(band ==
Green) {
1430 else if(band ==
Blue) {
1445 stretch.
AddPair(-DBL_MAX, 0.0);
1446 stretch.
AddPair(DBL_MAX, 255.0);
1465 for(
int line = 0; line < cube->
lineCount(); line++) {
1488 "Cannot stretch while the cube is still loading",
1492 QRect dataArea = QRect(buffer->
bufferXYRect().intersected(rect));
1495 for(
int y = dataArea.top();
1496 !dataArea.isNull() && y <= dataArea.bottom();
1500 for(
int x = dataArea.left(); x < dataArea.right(); x++) {
1520 double min,
double max) {
1524 for(
int line = 0; line < cube->
lineCount(); line++) {
1561 QRect rect,
double min,
double max) {
1562 QRect dataArea = QRect(buffer->
bufferXYRect().intersected(rect));
1567 for(
int y = dataArea.top(); !dataArea.isNull() && y <= dataArea.bottom(); y++) {
1569 hist.
AddData(&line.front() + (dataArea.left() - buffer->
bufferXYRect().left()), dataArea.width());
1578 std::string msg =
"Insufficient data Min [" + sMin +
"], Max [" + sMax +
"]";
1579 msg +=
" in the stretch area.";
void SetBasePosition(const int start_sample, const int start_line, const int start_band)
This method is used to set the base position of the shape buffer.
bool isRgbMode() const
Returns true if the dialog is displaying the RGB advanced stretches.
Reads and stores visible DN values.
void restoreRgbStretch(CubeStretch red, CubeStretch green, CubeStretch blue)
Restores a saved RGB stretch from the cube.
Cube display widget for certain Isis MDI applications.
ViewportBuffer * redBuffer()
Returns the red viewport buffer (Will be NULL if in Gray mode.)
void setAllBandStretches(Stretch stretch)
Sets a stretch for all bands.
This class is used to accumulate statistics on double arrays.
void updateHistogram(const Histogram &grayHist)
This calls setHistogram on the gray advanced stretches.
void AddData(const double *data, const unsigned int count)
Add an array of doubles to the accumulators and counters.
const std::vector< double > & getLine(int line)
Retrieves a line from the buffer.
double Percent(const double percent) const
Computes and returns the value at X percent of the histogram.
A single keyword-value pair.
PvlObjectIterator beginObject()
Returns the index of the beginning object.
void read(Blob &blob, const std::vector< PvlKeyword > keywords=std::vector< PvlKeyword >()) const
This method will read data from the specified Blob object.
CubeStretch getGrayStretch()
This returns the advanced stretch's stretch for gray.
@ Unknown
A type of error that cannot be classified as any of the other error types.
void updateStretch(CubeViewport *)
This calls setStretch on all applicable advanced stretches.
double * DoubleBuffer() const
Returns the value of the shape buffer.
double Maximum() const
Returns the absolute maximum double found in all data passed through the AddData method.
Container for cube-like labels.
void forgetStretches()
Resets all remembered stretches.
int Pairs() const
Returns the number of stretch pairs.
void setName(QString name)
Set the Stretch name.
Stores stretch information for a cube.
CubeStretch greenStretch() const
Return the green band stretch.
BigInt ValidPixels() const
Returns the total number of valid pixels processed.
Buffer for containing a three dimensional section of an image.
CubeStretch redStretch() const
Return the red band stretch.
CubeStretch getBluStretch()
This returns the advanced stretch's stretch for blue.
void stretchKnownGlobal()
List<Tool *> p This stretches to the global stretch.
QList< PvlObject >::iterator PvlObjectIterator
The counter for objects.
void restoreGrayStretch(CubeStretch stretch)
Restores a saved grayscale stretch from the cube.
CubeStretch blueStretch() const
Return the blue band stretch.
void updateHistograms(const Histogram &redHist, const Histogram &grnHist, const Histogram &bluHist)
This calls setHistogram on all of the advanced stretches.
This was called the Qisis MainWindow.
CubeStretch grayStretch() const
Return the gray band stretch.
void CopyPairs(const Stretch &other)
Copies the stretch pairs from another Stretch object, but maintains special pixel values.
int toInt(const QString &string)
Global function to convert from a string to an integer.
virtual void AddData(const double *data, const unsigned int count)
Add an array of doubles to the histogram counters.
double Minimum() const
Returns the absolute minimum double found in all data passed through the AddData method.
void AddPair(const double input, const double output)
Adds a stretch pair to the list of pairs.
void ClearPairs()
Clears the stretch pairs.
CubeStretch getRedStretch()
This returns the advanced stretch's stretch for red.
double BestMaximum(const double percent=99.5) const
This method returns the better of the absolute maximum or the Chebyshev maximum.
IO Handler for Isis Cubes.
Widget to display Isis cubes for qt apps.
QRect bufferXYRect()
Returns a rect, in screen pixels, of the area this buffer covers.
void enable(bool enable)
Sets the enabled state to enable.
Isis::Blob toBlob() const
Serialize the CubeStretch to a Blob.
bool deleteBlob(QString BlobName, QString BlobType)
This method will delete a blob label object from the cube as specified by the Blob type and name.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
void stretchGreen(const QString &string)
Apply stretch pairs to green bands.
void enableGrayMode(Stretch &grayStretch, Histogram &grayHist)
This displays a gray advanced stretch.
void updateForRGBMode(Stretch &redStretch, Histogram &redHist, Stretch &grnStretch, Histogram &grnHist, Stretch &bluStretch, Histogram &bluHist)
Update the stretch and histogram for all the bands for All BandId option.
void setBandNumber(int bandNumber)
Set the band number for the stretch.
ViewportBuffer * blueBuffer()
Returns the blue viewport buffer (Will be NULL if in Gray mode.)
bool isReadOnly() const
Test if the opened cube is read-only, that is write operations will fail if this is true.
PixelType pixelType() const
Container of a cube histogram.
double BestMinimum(const double percent=99.5) const
This method returns the better of the absolute minimum or the Chebyshev minimum.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
void write(Blob &blob, bool overwrite=true)
This method will write a blob of data (e.g.
This is free and unencumbered software released into the public domain.
bool hasEntireCube()
Method to see if the entire cube is in the buffer.
bool enabled()
Returns true if the advanced stretch is enabled.
Adds specific functionality to C++ strings.
ViewportBuffer * grayBuffer()
Returns the gray viewport buffer (Will be NULL if in RGB mode.)
CubeStretch getGrnStretch()
This returns the advanced stretch's stretch for green.
CubeStretch readCubeStretch(QString name="CubeStretch", const std::vector< PvlKeyword > keywords=std::vector< PvlKeyword >()) const
Read a Stretch from a cube.
void stretchGray(const QString &string)
Apply stretch pairs to gray band.
void stretchBlue(const QString &string)
Apply stretch pairs to blue bands.
ViewportBuffer * greenBuffer()
Returns the green viewport buffer (Will be NULL if in Gray mode.)
This is free and unencumbered software released into the public domain.
void reopen(QString access="r")
This method will reopen an isis sube for reading or reading/writing.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
double Input(const int index) const
Returns the value of the input side of the stretch pair at the specified index.
bool working()
This tests if queued actions exist in the viewport buffer.
void enableRgbMode(Stretch &redStretch, Histogram &redHist, Stretch &grnStretch, Histogram &grnHist, Stretch &bluStretch, Histogram &bluHist)
This displays RGB advanced stretches.
void stretchRed(const QString &string)
Apply stretch pairs to red bands.