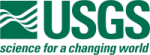 |
Isis 3 Programmer Reference
|
7 #include "UniversalGroundMap.h"
12 #include "CameraFactory.h"
13 #include "ImagePolygon.h"
15 #include "Longitude.h"
17 #include "Longitude.h"
18 #include "PolygonTools.h"
19 #include "Projection.h"
20 #include "RingPlaneProjection.h"
21 #include "TProjection.h"
22 #include "ProjectionFactory.h"
23 #include "SurfacePoint.h"
56 QString msg =
"Could not create camera or projection for [" +
59 realError.
append(firstError);
60 realError.
append(secondError);
137 double universalLat = lat.
degrees();
138 double universalLon = lon.
degrees();
164 double universalLat = lat.
degrees();
165 double universalLon = lon.
degrees();
322 bool allowEstimation) {
340 poly.
Polys()->clone());
342 geos::geom::Geometry *envelope = footprint->getEnvelope();
343 geos::geom::CoordinateSequence *coords = envelope->getCoordinates();
345 for (
unsigned int i = 0; i < coords->getSize(); i++) {
346 const geos::geom::Coordinate &coord = coords->getAt(i);
351 if (!minLat.
isValid() || minLat > coordLat)
353 if (!maxLat.
isValid() || maxLat < coordLat)
356 if (!minLon.
isValid() || minLon > coordLon)
358 if (!maxLon.
isValid() || maxLon < coordLon)
380 mappingGrp +=
PvlKeyword(
"LatitudeType",
"Planetocentric");
381 mappingGrp +=
PvlKeyword(
"LongitudeDomain",
"360");
382 mappingGrp +=
PvlKeyword(
"LongitudeDirection",
"PositiveEast");
385 mappingPvl += mappingGrp;
391 minLatDouble, maxLatDouble,
392 minLonDouble, maxLonDouble, mappingPvl);
401 if (mappingGrp.
hasKeyword(
"MinimumLatitude") &&
406 minLat =
Latitude(mappingGrp[
"MinimumLatitude"],
408 maxLat =
Latitude(mappingGrp[
"MaximumLatitude"],
410 minLon =
Longitude(mappingGrp[
"MinimumLongitude"],
412 maxLon =
Longitude(mappingGrp[
"MaximumLongitude"],
416 else if (allowEstimation && cube) {
446 int stepsPerLength = 20;
447 double aspectRatio = (double)lineCount / (
double)sampleCount;
448 double xStepSize = sampleCount / stepsPerLength;
449 double yStepSize = xStepSize * aspectRatio;
451 if (lineCount > sampleCount) {
452 aspectRatio = (double)sampleCount / (
double)lineCount;
453 yStepSize = lineCount / stepsPerLength;
454 xStepSize = yStepSize * aspectRatio;
457 double yWalked = 0.5;
460 for (
int i = 0; i < 3; i++) {
461 double xValue = 0.5 + ( i * (sampleCount / 2) );
463 while (yWalked <= lineCount) {
464 imagePoints.append( QPointF(xValue, yWalked) );
465 yWalked += yStepSize;
471 double xWalked = 0.5;
474 for (
int i = 0; i < 3; i++) {
475 double yValue = 0.5 + ( i * (lineCount / 2) );
477 while (xWalked <= sampleCount) {
478 imagePoints.append( QPointF(xWalked, yValue) );
479 xWalked += xStepSize;
485 double xDiagonalWalked = 0.5;
486 double yDiagonalWalked = 0.5;
487 xStepSize = sampleCount / stepsPerLength;
488 yStepSize = lineCount / stepsPerLength;
491 while ( (xDiagonalWalked <= sampleCount) && (yDiagonalWalked <= lineCount) ) {
492 imagePoints.append( QPointF(xDiagonalWalked, yDiagonalWalked) );
493 xDiagonalWalked += xStepSize;
494 yDiagonalWalked += yStepSize;
497 xDiagonalWalked = 0.5;
500 while ( (xDiagonalWalked <= sampleCount) && (yDiagonalWalked >= 0) ) {
501 imagePoints.append( QPointF(xDiagonalWalked, yDiagonalWalked) );
502 xDiagonalWalked += xStepSize;
503 yDiagonalWalked -= yStepSize;
506 foreach (QPointF imagePoint, imagePoints) {
507 if (tproj->
SetWorld(imagePoint.x(), imagePoint.y())) {
513 minLat = qMin(minLat, latResult);
518 maxLat = qMax(maxLat, latResult);
523 minLon = qMin(minLon, lonResult);
528 maxLon = qMax(maxLon, lonResult);
539 minLat < maxLat && minLon < maxLon);
virtual void SetBand(const int band)
Virtual method that sets the band number.
@ Degrees
Degrees are generally considered more human readable, 0-360 is one circle, however most math does not...
static Camera * Create(Cube &cube)
Creates a Camera object using Pvl Specifications.
ShapeModel * shape() const
Return the shape.
virtual QString fileName() const
Returns the opened cube's filename.
double Sample() const
Returns the current line value of the camera model or projection.
double UniversalLatitude() const
Returns the universal latitude of the camera model or projection.
A single keyword-value pair.
~UniversalGroundMap()
Destroys the UniversalGroundMap object.
double Resolution() const
This method returns the resolution for mapping world coordinates into projection coordinates.
This class is designed to encapsulate the concept of a Latitude.
virtual double UniversalLatitude()
This returns a universal latitude (planetocentric).
virtual bool SetImage(const double sample, const double line)
Sets the sample/line values of the image to get the lat/lon values.
bool SetGround(Latitude lat, Longitude lon)
Returns whether the lat/lon position was set successfully in the camera model or projection.
virtual double Sample() const
Returns the current sample number.
@ Unknown
A type of error that cannot be classified as any of the other error types.
ImagePolygon readFootprint() const
Read the footprint polygon for the Cube.
double RingLongitude() const
This returns a ring longitude with correct ring longitude direction and domain as specified in the la...
QString name() const
Gets the shape name.
ProjectionType projectionType() const
Returns an enum value for the projection type.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
Latitude GetLatitude() const
Return the body-fixed latitude for the surface point.
Create cube polygons, read/write polygons to blobs.
virtual bool SetUniversalGround(const double coord1, const double coord2)
This method is used to set the lat/lon or radius/azimuth (i.e.
virtual bool SetGround(Latitude latitude, Longitude longitude)
Sets the lat/lon values to get the sample/line values.
Isis::Projection * p_projection
The projection (if the image is projected)
void append(const IException &exceptionSource)
Appends the given exception (and its list of previous exceptions) to this exception's causational exc...
This class is designed to encapsulate the concept of a Longitude.
virtual Target * target() const
Returns a pointer to the target object.
virtual double WorldY() const
This returns the world Y coordinate provided SetGround, SetCoordinate, SetUniversalGround,...
bool InCube()
This returns true if the current Sample() or Line() value is outside of the cube (meaning the point m...
Base class for Map Projections of plane shapes.
Contains multiple PvlContainers.
virtual double UniversalLongitude() const
Returns the positive east, 0-360 domain longitude, in degrees, at the surface intersection point in t...
bool HasCamera()
Returns whether the ground map has a camera or not.
double RingRadius() const
This returns a radius.
Base class for Map TProjections.
virtual bool SetUniversalGround(const double latitude, const double longitude)
Sets the lat/lon values to get the sample/line values.
@ RingPlane
These projections are used to map ring planes.
@ CameraFirst
This is the default because cameras are projection-aware.
virtual double WorldX() const
This returns the world X coordinate provided SetGround, SetCoordinate, SetUniversalGround,...
bool HasProjection()
Returns whether the ground map has a projection or not.
IO Handler for Isis Cubes.
virtual bool SetWorld(const double x, const double y)
This method is used to set a world coordinate.
CameraPriority
This enum is used to define whether to use a camera or projection primarily, and which to fall back o...
Longitude GetLongitude() const
Return the body-fixed longitude for the surface point.
static Isis::Projection * CreateFromCube(Isis::Cube &cube)
This method is a helper method.
UniversalGroundMap(Cube &cube, CameraPriority priority=CameraFirst)
Constructs a UniversalGroundMap object from a cube.
double Resolution() const
Returns the resolution of the camera model or projection.
bool isValid() const
This indicates whether we have a legitimate angle stored or are in an unset, or invalid,...
virtual double PixelResolution()
Returns the pixel resolution at the current position in meters/pixel.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
virtual double UniversalLongitude()
This returns a universal longitude (positive east in 0 to 360 domain).
bool GroundRange(double &minlat, double &maxlat, double &minlon, double &maxlon, Pvl &pvl)
Computes the Ground Range.
bool SetUnboundGround(Latitude lat, Longitude lon)
Returns whether the lat/lon position was set successfully in the camera model or projection.
double degrees() const
Get the angle in units of Degrees.
bool SetUniversalGround(double lat, double lon)
Returns whether the lat/lon position was set successfully in the camera model or projection.
@ Triaxial
These projections are used to map triaxial and irregular-shaped bodies.
bool SetImage(double sample, double line)
Returns whether the sample/line postion was set successfully in the camera model or projection.
virtual double Line() const
Returns the current line number.
bool GroundRange(Cube *cube, Latitude &minLat, Latitude &maxLat, Longitude &minLon, Longitude &maxLon, bool allowEstimation=true)
Find the lat/lon range of the image.
double Line() const
Returns the current line value of the camera model or projection.
ProjectionType
This enum defines the subclasses of Projection supported in Isis.
This class defines a body-fixed surface point.
Isis::Camera * p_camera
The camera (if the image has a camera)
geos::geom::MultiPolygon * Polys()
Return a geos Multipolygon.
double UniversalLongitude() const
Returns the universal longitude of the camera model or projection.
This is free and unencumbered software released into the public domain.
virtual bool SetUnboundUniversalGround(const double coord1, const double coord2)
This method is used to set the lat/lon or radius/azimuth (i.e.
void SetBand(const int band)
Set the image band number.
virtual double UniversalLatitude() const
Returns the planetocentric latitude, in degrees, at the surface intersection point in the body fixed ...