File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
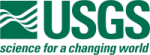 |
Isis 3 Programmer Reference
|
9 #include "VimsSkyMap.h"
18 #include "IException.h"
21 #include "LeastSquares.h"
22 #include "PolynomialBivariate.h"
23 #include "Preference.h"
24 #include "SpecialPixel.h"
64 QString stime = (QString) inst [
"NativeStartTime"];
65 QString intTime = stime.split(
".").first();
66 stime = stime.split(
".").last();
80 QString sampMode = QString((QString)inst [
"SamplingMode"]).toUpper();
83 int sampOffset = inst [
"XOffset"];
84 int lineOffset = inst [
"ZOffset"];
91 if (sampMode ==
"NORMAL") {
98 else if (sampMode ==
"HI-RES") {
108 string msg =
"Unsupported SamplingMode [" +
IString(sampMode) +
"]";
113 if (sampMode ==
"NORMAL") {
119 else if (sampMode ==
"HI-RES") {
128 string msg =
"Unsupported SamplingMode [" +
IString(sampMode) +
"]";
136 p_raMap[line][samp] = Isis::NULL8;
231 SpiceDouble lookC[3];
234 SpiceDouble unitLookC[3];
235 vhat_c(lookC, unitLookC);
248 if(ra < p_minRa || ra >
p_maxRa ||
253 double minDist = 9999.;
260 double mapRa =
p_raMap[line][samp];
261 if(mapRa == Isis::NULL8)
continue;
262 double mapDec =
p_decMap[line][samp];
263 if(mapDec == Isis::NULL8)
continue;
267 if(abs(mapRa - ra) > 180) {
268 if((ra - mapRa) > 0) {
269 mapRa = 360. + mapRa;
271 else if((ra - mapRa) < 0) {
272 mapRa = mapRa - 360.;
275 double dist = ((ra - mapRa) * (ra - mapRa)) +
276 ((dec - mapDec) * (dec - mapDec));
290 if(minDist >= 9999.)
return false;
301 vector<double> known(2);
303 for(
int line = minLine - 1; line < minLine + 2; line++) {
305 for(
int samp = minSamp - 1; samp < minSamp + 2; samp++) {
309 double mapRa =
p_raMap[line][samp];
310 double mapDec =
p_decMap[line][samp];
311 if((mapRa == Isis::NULL8) || (mapDec == Isis::NULL8))
continue;
316 if(abs(mapRa - ra) > 180) {
317 if((ra - mapRa) > 0) {
318 mapRa = 360. + mapRa;
320 else if((ra - mapRa) < 0) {
321 mapRa = mapRa - 360.;
331 if(sampLsq.
Knowns() < 3)
return false;
339 double inSamp = sampLsq.
Evaluate(known);
340 double inLine = lineLsq.
Evaluate(known);
380 v[0] = sin(theta) * cos(phi);
382 v[2] = sin(theta) * sin(phi) / -1.;
double p_maxDec
Maximum declination.
double p_uz
Distorted focal plane z, in millimeters.
void IgnoreProjection(bool ignore)
Set whether or not the camera should ignore the Projection.
const double HALFPI
The mathematical constant PI/2.
SpiceDouble p_etStart
Start ephemeris time.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
double p_focalPlaneX
Undistorted x value for the focal plane.
double p_uy
Distorted focal plane y, in millimeters.
int Solve(Isis::LeastSquares::SolveMethod method=SVD)
After all the data has been registered through AddKnown, invoke this method to solve the system of eq...
Camera * p_camera
The main camera to calculate distortions on.
double p_interlineDelay
InterlineDelayDuration keyword value from the instrument group of the labels, divided by 1000.
double p_decMap[64][64]
Declination map.
int ParentLines() const
Returns the number of lines in the parent alphacube.
double p_minRa
Minimum right ascension.
virtual bool SetImage(const double sample, const double line)
Sets the sample/line values of the image to get the lat/lon values.
int Knowns() const
The number of knowns (or times AddKnown was invoked) linear combination of the variables.
virtual bool SetSky(const double ra, const double dec)
Sets the sky position to the given ra and dec.
virtual iTime getClockTime(QString clockValue, int sclkCode=-1, bool clockTicks=false)
This converts the spacecraft clock ticks value (clockValue) to an iTime.
virtual double Declination()
Returns the declination angle (sky latitude).
Container for cube-like labels.
virtual bool SetFocalPlane(const double ux, const double uy, const double uz)
Compute ground position from focal plane coordinate.
double Evaluate(const std::vector< double > &input)
Invokes the BasisFunction Evaluate method.
double p_yPixSize
Y pixel size.
virtual double RightAscension()
Returns the right ascension angle (sky longitude).
double p_focalPlaneY
Undistorted y value for the focal plane.
double p_xBore
X boresight.
@ Traverse
Search child objects.
double p_yBore
Y boresight.
int ParentSamples() const
Returns the number of samples in the parent alphacube.
int p_swathLength
SwathLength keyword value from the instrument group of the labels.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
Nth degree Polynomial with two variables.
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
Contains multiple PvlContainers.
Generic least square fitting class.
double p_visExp
VIS exposure duration, divided by 1000.
double p_ux
Distorted focal plane x, in millimeters.
int p_swathWidth
SwathWidth keyword value from the instrument group of the labels.
double p_maxRa
Maximum right ascension.
int p_camSampOffset
Sample offset.
double p_raMap[64][64]
Right ascension map.
void Init(Pvl &lab)
Initialize vims sky model.
double toDouble(const QString &string)
Global function to convert from a string to a double.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
bool SetLookDirection(const double v[3])
Sets the look direction of the spacecraft.
Convert between undistorted focal plane and ra/dec coordinates.
double p_xPixSize
X pixel size.
double p_minDec
Minimum declination.
Adds specific functionality to C++ strings.
double p_irExp
IR exposure duration, divided by 1000.
void AddKnown(const std::vector< double > &input, double expected, double weight=1.0)
Invoke this method for each set of knowns.
QString p_channel
Channel keyword value from the instrument group of the labels.
int p_camLineOffset
Line offset.
void LookDirection(double v[3])
Determines the look direction in the camera coordinate system.
This is free and unencumbered software released into the public domain.