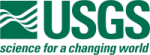 |
Isis 3 Programmer Reference
|
10 #include "VoyagerCamera.h"
16 #include "CameraDetectorMap.h"
17 #include "CameraFocalPlaneMap.h"
18 #include "CameraGroundMap.h"
19 #include "CameraSkyMap.h"
23 #include "NaifStatus.h"
24 #include "ReseauDistortionMap.h"
62 QString spacecraft = (QString)inst[
"SpacecraftName"];
63 QString instId = (QString)inst[
"InstrumentId"];
65 QString reseauFileName =
"";
68 if (spacecraft ==
"VOYAGER_1") {
74 reseauFileName +=
"1/reseaus/vg1";
76 if (instId ==
"NARROW_ANGLE_CAMERA") {
77 reseauFileName +=
"na";
81 else if (instId ==
"WIDE_ANGLE_CAMERA") {
82 reseauFileName +=
"wa";
87 QString msg =
"File does not appear to be a Voyager image. InstrumentId ["
88 + instId +
"] is invalid Voyager value.";
92 else if (spacecraft ==
"VOYAGER_2") {
98 reseauFileName +=
"2/reseaus/vg2";
100 if (instId ==
"NARROW_ANGLE_CAMERA") {
101 reseauFileName +=
"na";
105 else if (instId ==
"WIDE_ANGLE_CAMERA") {
106 reseauFileName +=
"wa";
111 QString msg =
"File does not appear to be a Voyager image. InstrumentId ["
112 + instId +
"] is invalid Voyager value.";
117 QString msg =
"File does not appear to be a Voyager image. SpacecraftName ["
118 + spacecraft +
"] is invalid Voyager value.";
129 reseauFileName =
"$voyager" + reseauFileName +
"MasterReseaus.pvl";
130 FileName masterReseaus(reseauFileName);
146 startTime.setUtc((QString)inst[
"StartTime"]);
190 double exposureDuration) {
191 pair<iTime, iTime> shuttertimes;
193 shuttertimes.second =
time - 2;
void SetDetectorOrigin(const double sample, const double line)
Set the detector origin.
virtual double exposureDuration() const
Return the exposure duration for the pixel that the camera is set to.
iTime time() const
Returns the ephemeris time in seconds which was used to obtain the spacecraft and sun positions.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
void print() const
Prints a string representation of this exception to stderr.
Convert between parent image coordinates and detector coordinates.
virtual std::pair< iTime, iTime > ShutterOpenCloseTimes(double time, double exposureDuration)
Returns the shutter open and close times.
SpiceInt naifIkCode() const
This returns the NAIF IK code to use when reading from instrument kernels.
Parse and return pieces of a time string.
File name manipulation and expansion.
QString m_instrumentNameLong
Full instrument name.
Container for cube-like labels.
int p_ckFrameId
"Camera-matrix" Kernel Frame ID
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
Generic class for Framing Cameras.
Distort/undistort focal plane coordinates.
Convert between undistorted focal plane and ground coordinates.
@ Traverse
Search child objects.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
void SetPixelPitch()
Reads the Pixel Pitch from the instrument kernel.
Contains multiple PvlContainers.
int p_spkTargetId
Spacecraft Kernel Target ID.
IO Handler for Isis Cubes.
QString m_spacecraftNameLong
Full spacecraft name.
Namespace for the standard library.
void LoadCache()
This loads the spice cache big enough for this image.
QString m_instrumentNameShort
Shortened instrument name.
Convert between distorted focal plane and detector coordinates.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
Convert between undistorted focal plane and ra/dec coordinates.
QString m_spacecraftNameShort
Shortened spacecraft name.
void SetFocalLength()
Reads the focal length from the instrument kernel.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....