File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
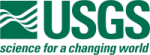 |
Isis 3 Programmer Reference
|
9 #include "DawnVirCamera.h"
20 #include <tnt/tnt_array2d_utils.h>
23 #include "CameraFocalPlaneMap.h"
24 #include "IException.h"
28 #include "LineScanCameraDetectorMap.h"
29 #include "LineScanCameraGroundMap.h"
30 #include "LineScanCameraSkyMap.h"
31 #include "NaifStatus.h"
32 #include "NumericalApproximation.h"
56 int procLevel = archive[
"ProcessingLevelId"];
61 QString channelId = inst[
"ChannelId"];
63 QString instMode = inst[
"InstrumentModeId"];
71 if (channelId ==
"VIS") {
73 virFrame = (hasArtCK) ? -203211 : -203221;
77 virFrame = (hasArtCK) ? -203213 : -203223;
90 PvlKeyword &frameParam = inst[
"FrameParameter"];
106 double sampleBoreSight =
getDouble(ikernKey);
109 double lineBoreSight =
getDouble(ikernKey);
140 #if defined(DUMP_INFO)
142 cout <<
"Total Records: " << cache.
Records() <<
"\n";
144 for (
int i = 0 ; i < cache.
Records() ; i++) {
146 string separator(
"");
147 for (
int f = 0 ; f < rec.
Fields() ; f++) {
148 cout << separator << (double) rec[f];
207 for (
int i = 0 ; i < text.size() ; i++) {
208 if ((text[i] >
' ') && (text[i] <=
'z')) ostr += text[i];
311 Table hktable(
"VIRHouseKeeping", filename);
316 for (
int i = 0; i < hktable.
Records(); i++) {
318 QString scet =
scrub(trec[
"ScetTimeClock"]);
319 QString shutterMode =
scrub(trec[
"ShutterStatus"]);
322 double mirrorSin = trec[
"MirrorSin"];
323 double mirrorCos = trec[
"MirrorCos"];
324 double scanElecDeg = atan(mirrorSin/mirrorCos) * dpr_c();
325 double optAng = ((scanElecDeg - 3.7996979) * 0.25/0.257812);
333 bool isDark = shutterMode.toLower() ==
"closed";
336 if ( ! isDark ) { angFit.
AddData(lineno, optAng); }
338 #if defined(DUMP_INFO)
339 cout <<
"Line(" << ((isDark) ?
"C): " :
"O): ") << i
340 <<
", OptAng(D): " << setprecision(12) << optAng * dpr_c()
341 <<
", MidExpTime(ET): " << lineMidTime
383 for (
unsigned int a = 0 ; a <
m_mirrorData.size() ; a++) {
393 mess <<
"Number housekeeping lines determined (" <<
m_lineRates.size()
394 <<
") is not equal to image lines(" <<
Lines() <<
")";
411 const int zeroFrame) {
434 Table quats(
"SpiceRotation", record);
435 int nfields = record.
Fields();
437 QString virId =
"DAWN_VIR_" + virChannel;
438 QString virZero = virId +
"_ZERO";
441 int nvals = nfields - 1;
444 SpiceDouble eulang[6] = { 0.0, 0.0, 0.0, 0.0, 0.0, 0.0 };
445 SpiceDouble xform[6][6], xform2[6][6];
447 SpiceDouble q_av[7], *av(&q_av[4]);
449 for (
int i = 0 ; i < nlines ; i++) {
450 int index = min(i, nlines-1);
459 eul2xf_c(eulang, 1, 2, 3, xform);
460 mxmg_c(xform, &state[0][0], 6, 6, 6, xform2);
463 xf2rav_c(xform2, m, av);
467 for (
int k = 0 ; k < nvals ; k++) {
472 record[nvals] = etTime;
477 mess <<
"Failed to get point state for line " << i+1;
488 int virZeroId =
getInteger(
"FRAME_" + virZero);
493 quats.
Label() += tdf;
500 SpiceDouble identity[3][3];
505 for (
int i = 0 ; i < 3 ; i++) {
506 for (
int j = 0 ; j < 3 ; j++) {
511 quats.
Label() += crot;
534 const QString &frame2,
535 const double &etTime)
541 sxform_c(frame1.toLatin1().data(), frame2.toLatin1().data(), etTime,
542 (SpiceDouble (*)[6]) state[0]);
548 pxform_c(frame1.toLatin1().data(), frame2.toLatin1().data(), etTime,
549 (SpiceDouble (*)[3]) rot[0]);
551 SpiceDouble av[3] = {0.0, 0.0, 0.0 };
552 rav2xf_c((SpiceDouble (*)[3]) rot[0], av,
553 (SpiceDouble (*)[6]) state[0]);
557 mess <<
"Could not get state rotation for Frame1 (" << frame1
558 <<
") to Frame2 (" << frame2 <<
") at time " << etTime;
583 QRegExp virCk(
"*dawn_vir_?????????_?.bc");
584 virCk.setPatternSyntax(QRegExp::Wildcard);
585 for (
int i = 0 ; i < cks.size() ; i++) {
586 if ( virCk.exactMatch(cks[i]) )
return (
true);
Convert between undistorted focal plane and ra/dec coordinates.
void SetDetectorOrigin(const double sample, const double line)
Set the detector origin.
double m_mirrorSin
Raw mirror sine value.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
int m_summing
Summing/binnning mode.
A single keyword-value pair.
Determine SPICE kernels defined in an ISIS file.
SpiceInt naifIkCode() const
This returns the NAIF IK code to use when reading from instrument kernels.
bool m_isDarkCurrent
If the line is dark current data.
PvlObject & Label()
The Table's label.
Parse and return pieces of a time string.
double m_scanLineEt
Center of line time in ET.
~DawnVirCamera()
Destructor.
void addValue(QString value, QString unit="")
Adds a value with units.
QString m_instrumentNameLong
Full instrument name.
virtual iTime getClockTime(QString clockValue, int sclkCode=-1, bool clockTicks=false)
This converts the spacecraft clock ticks value (clockValue) to an iTime.
TNT::Array2D< SpiceDouble > SMatrix
2-D buffer
virtual int SpkReferenceId() const
SPK Reference ID - J2000.
void SetDetectorSampleSumming(const double summing)
Set sample summing mode.
int Fields() const
Returns the number of fields that are currently in the record.
SpiceInt getInteger(const QString &key, int index=0)
This returns a value from the NAIF text pool.
Container for cube-like labels.
QStringList getKernelList(const QString &ktype="") const
Provide a list of all the kernels found.
Convert between parent image coordinates and detector coordinates.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
double m_opticalAngle
Optical angle in degrees.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
void MinimizeCache(DownsizeStatus status)
Set the downsize status to minimize cache.
std::vector< ScanMirrorInfo > m_mirrorData
vector of mirror info for each line
Table Cache(const QString &tableName)
Return a table with J2000 to reference rotations.
virtual int CkReferenceId() const
CK Reference ID - J2000.
double endTime() const
Return end time for the entire cube.
char m_slitMode
Slit mode of the instrument.
virtual SpiceRotation * instrumentRotation() const
Accessor method for the instrument rotation.
@ NearestEndpoint
Evaluate() returns the y-value of the nearest endpoint if a is outside of the domain.
@ Traverse
Search child objects.
void LoadCache(double startTime, double endTime, int size)
Cache J2000 rotation quaternion over a time range.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
int m_lineNum
The line the info is for.
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
void SetPixelPitch()
Reads the Pixel Pitch from the instrument kernel.
Contains multiple PvlContainers.
double lineStartTime(const double midExpTime) const
Return the start time for a given line exposure time.
@ No
Do not downsize the cache.
Camera model for both Danw VIR VIS and IR instruments.
double lineEndTime(const double midExpTime) const
Return the end time for a given line exposure time.
@ Double
The values in the field are 8 byte doubles.
Class for storing Table blobs information.
QString scrub(const QString &text) const
Scrubs a string coming out of the housekeeping table.
double exposureTime() const
Return the exposure time.
Generic class for Line Scan Cameras.
QString fileName() const
Returns the filename used to initialise the Pvl object.
Convert between undistorted focal plane and ground coordinates.
Distort/undistort focal plane coordinates.
double startTime() const
Return start time for the entire cube.
IO Handler for Isis Cubes.
QString m_spacecraftNameLong
Full spacecraft name.
virtual int CkFrameId() const
CK Frame ID - Instrument Code from spacit run on CK.
bool m_is1BCalibrated
is determined by Archive/ProcessingLevelId
double m_exposureTime
Line exposure time.
double m_scanRate
Line scan rate.
SMatrix getStateRotation(const QString &frame1, const QString &frame2, const double &et) const
Compute the state rotation at a given time for given frames.
double toDouble(const QString &string)
Global function to convert from a string to a double.
double scanLineTime() const
Return the line scan rate.
CameraFocalPlaneMap * FocalPlaneMap()
Returns a pointer to the CameraFocalPlaneMap object.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
void LoadCache()
This loads the spice cache big enough for this image.
QString m_instrumentNameShort
Shortened instrument name.
Convert between distorted focal plane and detector coordinates.
void AddData(const double x, const double y)
Add a datapoint to the set.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
double Evaluate(const double a, const ExtrapType &etype=ThrowError)
Calculates interpolated or extrapolated value of tabulated data set for given domain value.
double m_mirrorCos
Raw mirror cosine value.
std::vector< LineRateChange > m_lineRates
vector of timing info for each line
void readHouseKeeping(const QString &filename, double lineRate)
Read the VIR houskeeping table from cube.
int Lines() const
Returns the number of lines in the image.
SpiceDouble getDouble(const QString &key, int index=0)
This returns a value from the NAIF text pool.
int Records() const
Returns the number of records.
SpiceInt naifSpkCode() const
This returns the NAIF SPK code to use when reading from SPK kernels.
void SetFrame(int frameCode)
Change the frame to the given frame code.
LineScanCameraDetectorMap * DetectorMap()
Returns a pointer to the LineScanCameraDetectorMap object.
QString m_spacecraftNameShort
Shortened spacecraft name.
int hkLineCount() const
Returns number of housekeeping records found in the cube Table.
int pixelSumming() const
Return the pixel summing rate.
void SetFocalLength()
Reads the focal length from the instrument kernel.
NumericalApproximation provides various numerical analysis methods of interpolation,...
This is free and unencumbered software released into the public domain.
bool hasArticulationKernel(Pvl &label) const
determine if the CK articulation kernels are present/given
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
Container class for storing timing information for a section of an image.
Table getPointingTable(const QString &channelId, const int zeroFrame)
Compute the pointing table for each line.
Class for storing an Isis::Table's field information.