File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
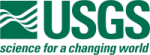 |
Isis 3 Programmer Reference
|
9 #include "RosettaVirtisCamera.h"
20 #include <tnt/tnt_array2d_utils.h>
23 #include "CameraFocalPlaneMap.h"
24 #include "IException.h"
28 #include "LineScanCameraDetectorMap.h"
29 #include "LineScanCameraGroundMap.h"
30 #include "LineScanCameraSkyMap.h"
31 #include "NaifStatus.h"
32 #include "NumericalApproximation.h"
60 QString mess =
"This data is apparently not from the VIRTIS instrument but "
66 int procLevel = inst[
"ProcessingLevelId"];
70 QString channelId = inst[
"ChannelId"];
72 QString instMode = inst[
"InstrumentModeId"];
82 if (channelId ==
"VIRTIS_M_VIS") {
84 virFrame = (hasArtCK) ? -226211 : -226112;
85 frameId =
"ROS_VIRTIS-M_VIS";
87 else if (channelId ==
"VIRTIS_M_IR") {
89 virFrame = (hasArtCK) ? -226213 : -226214;
90 frameId =
"ROS_VIRTIS-M_IR";
103 PvlKeyword &frameParam = inst[
"FrameParameter"];
128 double sampleBoreSight =
getDouble(ikernKey);
131 double lineBoreSight =
getDouble(ikernKey);
160 #if defined(DUMP_INFO)
162 cout <<
"Total Records: " << cache.
Records() <<
"\n";
164 for (
int i = 0 ; i < cache.
Records() ; i++) {
166 string separator(
"");
167 for (
int f = 0 ; f < rec.
Fields() ; f++) {
168 cout << separator << (double) rec[f];
303 std::vector<double> cacheTime;
304 Table hktable(
"VIRTISHouseKeeping", filename);
308 for (
int i = 0; i < hktable.
Records(); i++) {
310 QString scetString = trec[
"dataSCET"];
359 Table hktable(
"VIRTISHouseKeeping", filename);
364 for (
int i = 0; i < hktable.
Records(); i++) {
366 double scet = (double) trec[
"dataSCET"];
367 int shutterMode = (int) trec[
"Data Type__Shutter state"];
370 double mirrorSin = trec[
"M_MIRROR_SIN_HK"];
371 double mirrorCos = trec[
"M_MIRROR_COS_HK"];
372 double scanElecDeg = atan(mirrorSin/mirrorCos) * dpr_c();
373 double optAng = ((scanElecDeg - 3.7996979) * 0.25/0.257812);
381 bool isDark = (shutterMode == 1);
384 if ( ! isDark ) { angFit.
AddData(lineno, optAng); }
386 #if defined(DUMP_INFO)
387 cout <<
"Line(" << ((isDark) ?
"C): " :
"O): ") << i
388 <<
", OptAng(D): " << setprecision(12) << optAng * dpr_c()
389 <<
", MidExpTime(ET): " << lineMidTime
431 for (
unsigned int a = 0 ; a <
m_mirrorData.size() ; a++) {
441 mess <<
"Number housekeeping lines determined (" <<
m_lineRates.size()
442 <<
") is not equal to image lines(" <<
Lines() <<
")";
459 const int zeroFrame) {
482 Table quats(
"SpiceRotation", record);
483 int nfields = record.
Fields();
485 QString virZero = virChannel +
"_ZERO";
488 int nvals = nfields - 1;
491 SpiceDouble eulang[6] = { 0.0, 0.0, 0.0, 0.0, 0.0, 0.0 };
492 SpiceDouble xform[6][6], xform2[6][6];
494 SpiceDouble q_av[7], *av(&q_av[4]);
496 for (
int i = 0 ; i < nlines ; i++) {
497 int index = min(i, nlines-1);
506 eul2xf_c(eulang, 1, 2, 3, xform);
507 mxmg_c(xform, &state[0][0], 6, 6, 6, xform2);
510 xf2rav_c(xform2, m, av);
514 for (
int k = 0 ; k < nvals ; k++) {
519 record[nvals] = etTime;
524 mess <<
"Failed to get point state for line " << i+1;
535 int virZeroId =
getInteger(
"FRAME_" + virZero);
540 quats.
Label() += tdf;
547 SpiceDouble identity[3][3];
552 for (
int i = 0 ; i < 3 ; i++) {
553 for (
int j = 0 ; j < 3 ; j++) {
558 quats.
Label() += crot;
581 const QString &frame2,
582 const double &etTime)
588 sxform_c(frame1.toLatin1().data(), frame2.toLatin1().data(), etTime,
589 (SpiceDouble (*)[6]) state[0]);
595 pxform_c(frame1.toLatin1().data(), frame2.toLatin1().data(), etTime,
596 (SpiceDouble (*)[3]) rot[0]);
598 SpiceDouble av[3] = {0.0, 0.0, 0.0 };
599 rav2xf_c((SpiceDouble (*)[3]) rot[0], av,
600 (SpiceDouble (*)[6]) state[0]);
604 mess <<
"Could not get state rotation for Frame1 (" << frame1
605 <<
") to Frame2 (" << frame2 <<
") at time " << etTime;
630 QRegExp virCk(
"*ROS_VIRTIS_M_????_????_V?.BC");
631 virCk.setPatternSyntax(QRegExp::Wildcard);
632 for (
int i = 0 ; i < cks.size() ; i++) {
633 if ( virCk.exactMatch(cks[i]) )
return (
true);
double m_mirrorSin
Raw mirror sine value.
Convert between undistorted focal plane and ra/dec coordinates.
double endTime() const
Return end time for the entire cube.
void SetDetectorOrigin(const double sample, const double line)
Set the detector origin.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
int m_summing
Summing/binnning mode.
bool m_is1BCalibrated
is determined by Archive/ProcessingLevelId
A single keyword-value pair.
Determine SPICE kernels defined in an ISIS file.
SpiceInt naifIkCode() const
This returns the NAIF IK code to use when reading from instrument kernels.
PvlObject & Label()
The Table's label.
Parse and return pieces of a time string.
std::vector< LineRateChange > m_lineRates
vector of timing info for each line
void addValue(QString value, QString unit="")
Adds a value with units.
QString m_instrumentNameLong
Full instrument name.
virtual iTime getClockTime(QString clockValue, int sclkCode=-1, bool clockTicks=false)
This converts the spacecraft clock ticks value (clockValue) to an iTime.
void SetDetectorSampleSumming(const double summing)
Set sample summing mode.
int Fields() const
Returns the number of fields that are currently in the record.
SpiceInt getInteger(const QString &key, int index=0)
This returns a value from the NAIF text pool.
bool m_isDarkCurrent
If the line is dark current data.
Container for cube-like labels.
double m_exposureTime
Line exposure time.
QStringList getKernelList(const QString &ktype="") const
Provide a list of all the kernels found.
double m_opticalAngle
Optical angle in degrees.
~RosettaVirtisCamera()
Destructor.
Convert between parent image coordinates and detector coordinates.
double lineStartTime(const double midExpTime) const
Return the start time for a given line exposure time.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
void MinimizeCache(DownsizeStatus status)
Set the downsize status to minimize cache.
Table Cache(const QString &tableName)
Return a table with J2000 to reference rotations.
double m_scanLineEt
Center of line time in ET.
virtual SpiceRotation * instrumentRotation() const
Accessor method for the instrument rotation.
@ NearestEndpoint
Evaluate() returns the y-value of the nearest endpoint if a is outside of the domain.
@ Traverse
Search child objects.
SMatrix getStateRotation(const QString &frame1, const QString &frame2, const double &et) const
Compute the state rotation at a given time for given frames.
void LoadCache(double startTime, double endTime, int size)
Cache J2000 rotation quaternion over a time range.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
double exposureTime() const
Return the exposure time.
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
void SetPixelPitch()
Reads the Pixel Pitch from the instrument kernel.
Contains multiple PvlContainers.
@ No
Do not downsize the cache.
Camera model for both Rosetta VIRTIS-M instruments.
void readSCET(const QString &filename)
For calibrated VIRTIS-M images, read the SCET values from the cube.
TNT::Array2D< SpiceDouble > SMatrix
2-D buffer
QString instrumentId()
This method returns the InstrumentId as it appears in the cube.
@ Double
The values in the field are 8 byte doubles.
virtual int CkFrameId() const
CK Frame ID - Instrument Code from spacit run on CK.
Class for storing Table blobs information.
Generic class for Line Scan Cameras.
QString fileName() const
Returns the filename used to initialise the Pvl object.
std::vector< ScanMirrorInfo > m_mirrorData
vector of mirror info for each line
Convert between undistorted focal plane and ground coordinates.
int m_lineNum
The line the info is for.
Distort/undistort focal plane coordinates.
IO Handler for Isis Cubes.
QString m_spacecraftNameLong
Full spacecraft name.
double m_mirrorCos
Raw mirror cosine value.
virtual int CkReferenceId() const
CK Reference ID - J2000.
char m_slitMode
Slit mode of the instrument.
double toDouble(const QString &string)
Global function to convert from a string to a double.
CameraFocalPlaneMap * FocalPlaneMap()
Returns a pointer to the CameraFocalPlaneMap object.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
int pixelSumming() const
Return the pixel summing rate.
void LoadCache()
This loads the spice cache big enough for this image.
double scanLineTime() const
Return the line scan rate.
QString m_instrumentNameShort
Shortened instrument name.
Convert between distorted focal plane and detector coordinates.
void AddData(const double x, const double y)
Add a datapoint to the set.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
double Evaluate(const double a, const ExtrapType &etype=ThrowError)
Calculates interpolated or extrapolated value of tabulated data set for given domain value.
double lineEndTime(const double midExpTime) const
Return the end time for a given line exposure time.
int Lines() const
Returns the number of lines in the image.
SpiceDouble getDouble(const QString &key, int index=0)
This returns a value from the NAIF text pool.
double startTime() const
Return start time for the entire cube.
int Records() const
Returns the number of records.
SpiceInt naifSpkCode() const
This returns the NAIF SPK code to use when reading from SPK kernels.
void SetFrame(int frameCode)
Change the frame to the given frame code.
LineScanCameraDetectorMap * DetectorMap()
Returns a pointer to the LineScanCameraDetectorMap object.
QString m_spacecraftNameShort
Shortened spacecraft name.
int hkLineCount() const
Returns number of housekeeping records found in the cube Table.
Table getPointingTable(const QString &channelId, const int zeroFrame)
Compute the pointing table for each line.
double m_scanRate
Line scan rate.
void SetFocalLength()
Reads the focal length from the instrument kernel.
NumericalApproximation provides various numerical analysis methods of interpolation,...
virtual int SpkReferenceId() const
SPK Reference ID - J2000.
bool hasArticulationKernel(Pvl &label) const
determine if the CK articulation kernels are present/given
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
Container class for storing timing information for a section of an image.
void readHouseKeeping(const QString &filename, double lineRate)
Read the VIRTIS houskeeping table from cube.
Class for storing an Isis::Table's field information.