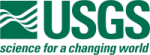 |
Isis 3 Programmer Reference
|
2 #include "SunShadowTool.h"
4 #include <QApplication>
12 #include <QMessageBox>
14 #include <QStackedWidget>
15 #include <QTableWidget>
16 #include <QToolButton>
23 #include "Longitude.h"
24 #include "MdiCubeViewport.h"
25 #include "Projection.h"
26 #include "SurfacePoint.h"
53 "Start\nLatitude:Start\nLongitude:End\nLatitude:End\nLongitude",
54 "Ground Range", -1, Qt::Horizontal,
55 "Start Latitude/Longitude to End Latitude/Longitude");
57 "Start\nSample:Start\nLine:End\nSample:End\nLine",
58 "Pixel Range", -1, Qt::Horizontal,
59 "Start Sample/Line to End Sample/Line");
65 "Incidence Angle (degrees)");
67 "Incidence Angle (radians)");
74 connect(
this, SIGNAL(viewportChanged()),
94 action->setIcon(QPixmap(
toolIconDir() +
"/sunshadow.png"));
95 action->setToolTip(
"Sun Shadow (U)");
96 action->setShortcut(Qt::Key_U);
99 "<b>Function:</b> Calculate heights or depths of features in the active "
100 "viewport given the measurement of a shadow. The shadow measurement "
101 "should originate from the top of the feature and end when the shadow "
103 "<p><b>Shortcut:</b> U</p> ";
104 action->setWhatsThis(text);
120 QToolButton *showTableButton =
new QToolButton(hbox);
121 showTableButton->setText(
"Table");
122 showTableButton->setToolTip(
"Record Measurement Data in Table");
124 "<b>Function:</b> This button will bring up a table that will record "
125 "the starting and ending points of the line, along with the calculated "
126 "values for the two points on the image. To measure a shadow, "
127 "click on the first point and releasing the mouse at the second point."
128 "\n<p><b>Shortcut:</b> CTRL+M</p>";
129 showTableButton->setWhatsThis(text);
130 showTableButton->setShortcut(Qt::CTRL + Qt::Key_M);
131 connect(showTableButton, SIGNAL(clicked()),
m_tableWin, SLOT(showTable()));
132 connect(showTableButton, SIGNAL(clicked()),
m_tableWin, SLOT(syncColumns()));
133 connect(showTableButton, SIGNAL(clicked()),
m_tableWin, SLOT(
raise()));
134 showTableButton->setEnabled(
true);
140 text =
"<b>Function: </b> Shows the height of the shadow drawn on "
156 QHBoxLayout *layout =
new QHBoxLayout(hbox);
157 layout->setMargin(0);
161 layout->addWidget(showTableButton);
162 layout->addStretch(1);
163 hbox->setLayout(layout);
199 painter->setPen(QPen(Qt::red));
200 painter->drawLine(QPoint(vpStartX, vpStartY), QPoint(vpEndX, vpEndY));
237 bool adjustLine =
true;
319 ASSERT(row < m_tableWin->table()->rowCount());
440 QTableWidgetItem *item =
new QTableWidgetItem(
"");
444 QAbstractItemView::PositionAtBottom);
493 QVector3D sunDirection;
497 double sunPosition[3];
501 cam->
radii(targetRadii);
503 double origin[3] = {0.0, 0.0, 0.0};
504 SpiceBoolean surfptSuccess;
506 double naifVectorFromSunToP1[3] = {0.0, 0.0, 0.0};
508 surfpt_c(origin, sunPosition, targetRadii[0].kilometers(),
509 targetRadii[1].kilometers(), targetRadii[2].kilometers(),
510 naifVectorFromSunToP1, &surfptSuccess);
511 success = surfptSuccess;
514 sunDirection = QVector3D(
515 naifVectorFromSunToP1[0] * 1000.0,
516 naifVectorFromSunToP1[1] * 1000.0,
517 naifVectorFromSunToP1[2] * 1000.0).normalized();
562 switch (displayUnits) {
591 bool hasCamera =
true;
593 hasCamera = activeViewport &&
double kilometers() const
Get the distance in kilometers.
void radii(Distance r[3]) const
Returns the radii of the body in km.
@ Degrees
Degrees are generally considered more human readable, 0-360 is one circle, however most math does not...
virtual void sunPosition(double p[3]) const
Fills the input vector with sun position information, in either body-fixed or J2000 reference frame a...
Cube display widget for certain Isis MDI applications.
QTableWidget * table() const
Returns the table.
@ Pixels
The distance is being specified in pixels.
virtual bool SetImage(const double sample, const double line)
Sets the sample/line values of the image to get the lat/lon values.
QString name() const
Returns the name of the file excluding the path and the attributes in the file name.
File name manipulation and expansion.
Latitude GetLatitude() const
Return the body-fixed latitude for the surface point.
Units
This is a list of available units to access and store Distances in.
@ SolarRadii
"Solar radius is a unit of distance used to express the size of stars in astronomy equal to the curre...
SurfacePoint GetSurfacePoint() const
Returns the surface point (most efficient accessor).
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
void addToTable(bool setOn, const QString &heading, const QString &menuText="", int insertAt=-1, Qt::Orientation o=Qt::Horizontal, QString toolTip="")
Adds a new column to the table when a new curve is added to the plot.
Distance measurement, usually in meters.
void setTrackListItems(bool track=false)
If this property is true, the class will keep track of the checked/unchecked items in the dock area w...
@ Kilometers
The distance is being specified in kilometers.
Displacement is a signed length, usually in meters.
void cubeToViewport(double sample, double line, int &x, int &y) const
Turns a cube into a viewport.
@ Meters
The distance is being specified in meters.
double meters() const
Get the displacement in meters.
int toInt(const QString &string)
Global function to convert from a string to an integer.
bool isValid() const
Test if this distance has been initialized or not.
a subclass of the qisis mainwindow, tablemainwindow handles all of the table tasks.
Defines an angle and provides unit conversions.
virtual double IncidenceAngle() const
Returns the incidence angle in degrees.
Longitude GetLongitude() const
Return the body-fixed longitude for the surface point.
const double Null
Value for an Isis Null pixel.
Camera * camera()
Return a camera associated with the cube.
bool isValid() const
This indicates whether we have a legitimate angle stored or are in an unset, or invalid,...
void setStatusMessage(QString message)
sets the status message in the lower lefthand corner of the window.
double meters() const
Get the distance in meters.
double degrees() const
Get the angle in units of Degrees.
void viewportToCube(int x, int y, double &sample, double &line) const
Turns a viewport into a cube.
double SunAzimuth()
Returns the Sun Azimuth.
This class defines a body-fixed surface point.
QString path() const
Returns the path of the file name.
This is free and unencumbered software released into the public domain.
double radians() const
Convert an angle to a double.