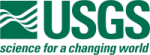 |
Isis 3 Programmer Reference
|
9 #include "MdiCubeViewport.h"
10 #include "Projection.h"
11 #include "RingPlaneProjection.h"
12 #include "SpecialPixel.h"
14 #include "TProjection.h"
15 #include "ViewportBuffer.h"
16 #include "WarningWidget.h"
118 if(cvp == NULL)
return;
120 if(p.x() >= 0 && p.x() < cvp->width() &&
121 p.y() >= 0 && p.y() < cvp->height()) {
153 if((sample < 0.5) || (line < 0.5) ||
159 int isamp = (int)(sample + 0.5);
165 int iline = (int)(line + 0.5);
184 p_latLabel->setText(QString(
"DEC %1").arg(lat));
185 p_lonLabel->setText(QString(
"RA %1").arg(lon));
188 p_latLabel->setText(QString(
"Lat %1").arg(lat));
189 p_lonLabel->setText(QString(
"Lon %1").arg(lon));
197 p_latLabel->setText(QString(
"Rad %1").arg(rad));
198 p_lonLabel->setText(QString(
"Lon %1").arg(lon));
207 else if(cvp->
camera() != NULL) {
215 p_latLabel->setText(QString(
"Lat %1").arg(lat));
216 p_lonLabel->setText(QString(
"Lon %1").arg(lon));
222 p_latLabel->setText(QString(
"Rad %1").arg(rad));
223 p_lonLabel->setText(QString(
"Lon %1").arg(lon));
251 if(p.x() >= rect.left() && p.x() <= rect.right() &&
252 p.y() >= rect.top() && p.y() <= rect.bottom()) {
253 const int bufX = p.x() - rect.left();
254 const int bufY = p.y() - rect.top();
275 if(p.x() >= rRect.left() && p.x() < rRect.right() &&
276 p.y() >= rRect.top() && p.y() < rRect.bottom()) {
277 const int rBufX = p.x() - rRect.left();
278 const int rBufY = p.y() - rRect.top();
294 if(p.x() >= gRect.left() && p.x() < gRect.right() &&
295 p.y() >= gRect.top() && p.y() < gRect.bottom()) {
296 const int gBufX = p.x() - gRect.left();
297 const int gBufY = p.y() - gRect.top();
313 if(p.x() >= bRect.left() && p.x() < bRect.right() &&
314 p.y() >= bRect.top() && p.y() < bRect.bottom()) {
315 const int bBufX = p.x() - bRect.left();
316 const int bBufY = p.y() - bRect.top();
349 QPoint p =
cubeViewport()->viewport()->mapFromGlobal(QCursor::pos());
350 if(p.x() < 0)
return;
351 if(p.y() < 0)
return;
352 if(p.x() >=
cubeViewport()->viewport()->width())
return;
353 if(p.y() >=
cubeViewport()->viewport()->height())
return;
382 QStatusBar *TrackTool::getStatusBar(
void) {
int cubeLines() const
Return the number of lines in the cube.
QString PixelToString(double d)
Takes a double pixel value and returns the name of the pixel type as a string.
Reads and stores visible DN values.
Cube display widget for certain Isis MDI applications.
ViewportBuffer * redBuffer()
Returns the red viewport buffer (Will be NULL if in Gray mode.)
ShapeModel * shape() const
Return the shape.
const std::vector< double > & getLine(int line)
Retrieves a line from the buffer.
virtual bool SetImage(const double sample, const double line)
Sets the sample/line values of the image to get the lat/lon values.
double RingLongitude() const
This returns a ring longitude with correct ring longitude direction and domain as specified in the la...
virtual double Longitude() const
This returns a longitude with correct longitude direction and domain as specified in the label object...
QString name() const
Gets the shape name.
ProjectionType projectionType() const
Returns an enum value for the projection type.
virtual Target * target() const
Returns a pointer to the target object.
Base class for Map Projections of plane shapes.
virtual double UniversalLongitude() const
Returns the positive east, 0-360 domain longitude, in degrees, at the surface intersection point in t...
virtual double Latitude() const
This returns a latitude with correct latitude type as specified in the label object.
double RingRadius() const
This returns a radius.
Base class for Map TProjections.
int cubeSamples() const
Return the number of samples in the cube.
virtual bool SetWorld(const double x, const double y)
This method is used to set a world coordinate.
QRect bufferXYRect()
Returns a rect, in screen pixels, of the area this buffer covers.
ViewportBuffer * blueBuffer()
Returns the blue viewport buffer (Will be NULL if in Gray mode.)
double meters() const
Get the distance in meters.
@ Triaxial
These projections are used to map triaxial and irregular-shaped bodies.
void viewportToCube(int x, int y, double &sample, double &line) const
Turns a viewport into a cube.
bool IsSky() const
Returns true if projection is sky and false if it is land.
Adds specific functionality to C++ strings.
ViewportBuffer * grayBuffer()
Returns the gray viewport buffer (Will be NULL if in RGB mode.)
ProjectionType
This enum defines the subclasses of Projection supported in Isis.
ViewportBuffer * greenBuffer()
Returns the green viewport buffer (Will be NULL if in Gray mode.)
This is free and unencumbered software released into the public domain.
QString ToQt() const
Retuns the object string as a QString.
Distance LocalRadius() const
Returns the local radius at the intersection point.
Projection * projection() const
virtual double UniversalLatitude() const
Returns the planetocentric latitude, in degrees, at the surface intersection point in the body fixed ...
bool working()
This tests if queued actions exist in the viewport buffer.