File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
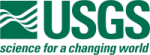 |
Isis 3 Programmer Reference
|
9 #include "VimsGroundMap.h"
17 #include "BasisFunction.h"
19 #include "Constants.h"
22 #include "IException.h"
26 #include "LeastSquares.h"
27 #include "Longitude.h"
28 #include "PolynomialBivariate.h"
29 #include "Preference.h"
30 #include "SpecialPixel.h"
42 VimsGroundMap::VimsGroundMap(
Camera *parent,
Pvl &lab) :
53 IString msg =
"The Vims ground map does not understand cubes that "
54 "initially have more than 64 lines or 64 samples.";
123 QString stime = (QString) inst [
"NativeStartTime"];
124 QString intTime = stime.split(
".").first();
125 stime = stime.split(
".").last();
138 QString sampMode = QString((QString)inst [
"SamplingMode"]).toUpper();
141 int sampOffset = inst [
"XOffset"];
142 int lineOffset = inst [
"ZOffset"];
149 if (sampMode ==
"NORMAL") {
156 else if (sampMode ==
"HI-RES") {
166 string msg =
"Unsupported SamplingMode [" +
IString(sampMode) +
"]";
171 if (sampMode ==
"NORMAL") {
177 else if (sampMode ==
"HI-RES") {
186 string msg =
"Unsupported SamplingMode [" +
IString(sampMode) +
"]";
223 if (xyz[0] !=
Null && xyz[1] !=
Null && xyz[2] !=
Null) {
224 p_xyzMap[line][samp].setX(xyz[0]);
225 p_xyzMap[line][samp].setY(xyz[1]);
226 p_xyzMap[line][samp].setZ(xyz[2]);
235 if (xyz[0] < p_minX) p_minX = xyz[0];
236 if (xyz[0] > p_maxX) p_maxX = xyz[0];
237 if (xyz[1] < p_minY) p_minY = xyz[1];
238 if (xyz[1] > p_maxY) p_maxY = xyz[1];
239 if (xyz[2] < p_minZ) p_minZ = xyz[2];
240 if (xyz[2] > p_maxZ) p_maxZ = xyz[2];
255 if (abs(abs(p_minZ) - radii[2].
kilometers()) < 1.0) {
256 p_minZ = radii[2].
kilometers() * (int(abs(p_minZ) / p_minZ));
258 if (abs(abs(p_maxZ) - radii[2].kilometers()) < 1.0) {
259 p_maxZ = radii[2].
kilometers() * (int(abs(p_maxZ) / p_maxZ));
319 SpiceDouble lookC[3];
322 SpiceDouble unitLookC[3];
323 vhat_c(lookC, unitLookC);
363 if (p_minX == DBL_MAX || p_maxX == -DBL_MAX ||
364 p_minY == DBL_MAX || p_maxY == -DBL_MAX ||
365 p_minZ == DBL_MAX || p_maxZ == -DBL_MAX)
return false;
373 double xCheck = radius * 0.001 * cos(lon.
radians());
374 double yCheck = radius * 0.001 * sin(lon.
radians());
387 if (pB[0] < p_minX || pB[0] > p_maxX ||
388 pB[1] < p_minY || pB[1] > p_maxY ||
389 pB[2] < p_minZ || pB[2] > p_maxZ)
return false;
396 double minDist = DBL_MAX;
404 if (p_xyzMap[line][samp].isNull())
continue;
407 QVector3D deltaXyz = xyz - p_xyzMap[line][samp];
408 if (deltaXyz.length() < minDist) {
409 minDist = deltaXyz.length();
421 if (minDist >= DBL_MAX)
return false;
432 vector<double> knownXyz(4);
435 for (
int line = minLine - 1; line < minLine + 2; line++) {
437 for (
int samp = minSamp - 1; samp < minSamp + 2; samp++) {
440 if (p_xyzMap[line][samp].isNull())
continue;
442 knownXyz[0] = p_xyzMap[line][samp].x();
443 knownXyz[1] = p_xyzMap[line][samp].y();
444 knownXyz[2] = p_xyzMap[line][samp].z();
446 sampXyzLsq.
AddKnown(knownXyz, samp + 1);
447 lineXyzLsq.
AddKnown(knownXyz, line + 1);
451 if (sampXyzLsq.
Knowns() < 4)
return false;
457 knownXyz[0] = xyz.x();
458 knownXyz[1] = xyz.y();
459 knownXyz[2] = xyz.z();
461 double inSamp = sampXyzLsq.
Evaluate(knownXyz);
462 double inLine = lineXyzLsq.
Evaluate(knownXyz);
520 v[0] = sin(theta) * cos(phi);
522 v[2] = sin(theta) * sin(phi) / -1.;
double kilometers() const
Get the distance in kilometers.
void radii(Distance r[3]) const
Returns the radii of the body in km.
double p_uy
Distorted focal plane y, in millimeters.
void IgnoreProjection(bool ignore)
Set whether or not the camera should ignore the Projection.
const double HALFPI
The mathematical constant PI/2.
ShapeModel * shape() const
Return the shape.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
int Solve(Isis::LeastSquares::SolveMethod method=SVD)
After all the data has been registered through AddKnown, invoke this method to solve the system of eq...
double p_focalPlaneY
Camera's y focal plane coordinate.
double p_irExp
IR exposure duration, divided by 1000.
int ParentLines() const
Returns the number of lines in the parent alphacube.
virtual bool SetGround(const Latitude &lat, const Longitude &lon)
Compute undistorted focal plane coordinate from ground position.
This class is designed to encapsulate the concept of a Latitude.
bool HasSurfaceIntersection() const
Returns if the last call to either SetLookDirection or SetUniversalGround had a valid intersection wi...
int p_camLineOffset
Line offset.
virtual bool SetImage(const double sample, const double line)
Sets the sample/line values of the image to get the lat/lon values.
int Knowns() const
The number of knowns (or times AddKnown was invoked) linear combination of the variables.
virtual bool SetFocalPlane(const double ux, const double uy, const double uz)
Compute ground position from focal plane coordinate.
Generic linear equation class.
SpiceDouble p_etStart
Start ephemeris time.
virtual iTime getClockTime(QString clockValue, int sclkCode=-1, bool clockTicks=false)
This converts the spacecraft clock ticks value (clockValue) to an iTime.
int p_swathLength
SwathLength keyword value from the instrument group of the labels.
double p_yPixSize
Y pixel size.
QString name() const
Gets the shape name.
Container for cube-like labels.
Latitude GetLatitude() const
Return the body-fixed latitude for the surface point.
double Evaluate(const std::vector< double > &input)
Invokes the BasisFunction Evaluate method.
void Init(Pvl &lab)
Initialize vims camera model.
double p_focalPlaneX
Camera's x focal plane coordinate.
virtual ~VimsGroundMap()
Destroys the VimsGroundMap object.
Distance measurement, usually in meters.
This class is designed to encapsulate the concept of a Longitude.
Convert between undistorted focal plane and ground coordinates.
virtual Target * target() const
Returns a pointer to the target object.
@ Traverse
Search child objects.
int ParentSamples() const
Returns the number of samples in the parent alphacube.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
Contains multiple PvlContainers.
Generic least square fitting class.
double p_visExp
VIS exposure duration, divided by 1000.
int p_swathWidth
SwathWidth keyword value from the instrument group of the labels.
double p_interlineDelay
InterlineDelayDuration keyword value from the instrument group of the labels, divided by 1000.
double p_xPixSize
X pixel size.
double p_uz
Distorted focal plane z, in millimeters.
void LookDirection(double v[3])
Determines the look direction in the camera coordinate system.
Longitude GetLongitude() const
Return the body-fixed longitude for the surface point.
const double Null
Value for an Isis Null pixel.
double p_xBore
X boresight.
double toDouble(const QString &string)
Global function to convert from a string to a double.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
bool SetLookDirection(const double v[3])
Sets the look direction of the spacecraft.
QString p_channel
Channel keyword value from the instrument group of the labels.
double degrees() const
Get the angle in units of Degrees.
double p_yBore
Y boresight.
void Coordinate(double p[3]) const
Returns the x,y,z of the surface intersection in BodyFixed km.
Adds specific functionality to C++ strings.
void AddKnown(const std::vector< double > &input, double expected, double weight=1.0)
Invoke this method for each set of knowns.
double p_ux
Distorted focal plane x, in millimeters.
int p_camSampOffset
Sample offset.
This class defines a body-fixed surface point.
This is free and unencumbered software released into the public domain.
Distance LocalRadius() const
Returns the local radius at the intersection point.
double radians() const
Convert an angle to a double.