File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
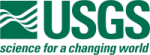 |
Isis 3 Programmer Reference
|
9 #include "AdvancedTrackTool.h"
12 #include <QApplication>
14 #include <QListIterator>
17 #include <QMessageBox>
18 #include <QPushButton>
19 #include <QScrollArea>
21 #include <QTableWidget>
22 #include <QTableWidgetItem>
24 #include <QVBoxLayout>
28 #include "CameraDistortionMap.h"
29 #include "CameraFocalPlaneMap.h"
32 #include "Longitude.h"
33 #include "MdiCubeViewport.h"
34 #include "Projection.h"
35 #include "RingPlaneProjection.h"
36 #include "SerialNumber.h"
37 #include "SpecialPixel.h"
38 #include "TableMainWindow.h"
40 #include "TProjection.h"
41 #include "TrackingTable.h"
46 #define FLOAT_MIN -16777215
61 p_action->setShortcut(Qt::CTRL + Qt::Key_T);
62 p_action->setWhatsThis(
"<b>Function: </b> Opens the Advanced Tracking Tool \
63 window. This window will track sample/line positions,\
64 lat/lon positions, and many other pieces of \
65 information. All of the data in the window can be \
66 saved to a text file. <p><b>Shortcut: </b> Ctrl+T</p>");
77 for (iter = checkBoxItems.begin(); iter != checkBoxItems.end(); ++iter) {
79 QString header = currentList[0];
80 QString menuText = currentList[2];
81 QString toolTip = currentList[3];
83 if (currentList[1] == QString(
"true")) {
90 if (toolTip != QString(
"")) {
92 -1, Qt::Horizontal, toolTip);
104 for(
int r = 0; r < 10; r++) {
107 QTableWidgetItem *item =
new QTableWidgetItem(
"");
114 recordAction->setShortcut(Qt::Key_R);
115 parent->addAction(recordAction);
116 connect(recordAction, SIGNAL(triggered()),
this, SLOT(
record()));
118 " --- Double click on a cell to enable crtl+c (copy) and"
123 QMenu *helpMenu = menuBar->addMenu(
"&Help");
125 help->setText(
"&Tool Help");
126 help->setShortcut(Qt::CTRL + Qt::Key_H);
127 connect(help, SIGNAL(triggered()),
this, SLOT(
helpDialog()));
128 helpMenu->addAction(help);
145 if(e->type() == QEvent::Show) {
153 else if(e->type() == QEvent::Hide) {
156 return Tool::eventFilter(o, e);
247 for (iter = checkBoxItems.begin(); iter != checkBoxItems.end(); ++iter) {
251 for (headerIter = splitHeader.begin(); headerIter != splitHeader.end(); ++headerIter) {
252 QString header = *headerIter;
253 if (header.toLower() == keyword.toLower()) {
259 IString msg =
"Header [" + keyword +
"] not found; make sure spelling is correct";
274 int isample = int (sample + 0.5);
275 int iline = int (line + 0.5);
292 QTableWidgetItem *item =
new QTableWidgetItem(
"");
304 if(sample < 0.5)
return;
305 if(line < 0.5)
return;
307 if(line > cvp->
cubeLines() + 0.5)
return;
324 QString fnamePath = fname.
path();
325 QString fnameName = fname.
name();
333 if((sample < 0.5) || (line < 0.5) ||
342 QString p = grayPixel;
347 QString p = redPixel;
352 if(cvp->
camera() != NULL) {
360 setText(QString::number(lat,
'f', 15));
362 setText(QString::number(lon,
'f', 15));
364 setText(QString::number(radius,
'f', 15));
372 while(lon < 0.0) lon += 360.0;
377 setText(QString::number(lat,
'f', 15));
379 setText(QString::number(lon,
'f', 15));
405 setText(QString::number(obliquePRes));
423 Angle phaseAngle, incidenceAngle, emissionAngle;
424 bool bSuccess =
false;
428 setText(QString::number(incidenceAngle.
degrees()));
430 setText(QString::number(emissionAngle.
degrees()));
443 setText(QString::number(northAzi));
452 setText(QString::number(sunAzi));
461 setText(QString::number(spacecraftAzi));
470 setText(QString::number(solarLon));
473 setText(QString::number(slantDistance));
476 setText(QString::number(lst));
483 setText(QString::number(undistortedFocalPlaneX));
486 setText(QString::number(undistortedFocalPlaneY));
489 setText(QString::number(undistortedFocalPlaneZ));
493 double distortedFocalPlaneX = focalPlaneMap->
FocalPlaneX();
495 setText(QString::number(distortedFocalPlaneX));
496 double distortedFocalPlaneY = focalPlaneMap->
FocalPlaneY();
498 setText(QString::number(distortedFocalPlaneY));
509 setText(QString::number(time.
Et(),
'f', 15));
510 QString time_utc = time.
UTC();
533 while(wlon < 0.0) wlon += 360.0;
534 if (tproj->
IsSky()) {
537 setText(QString::number(lon,
'f', 15));
539 setText(QString::number(lat,
'f', 15));
544 setText(QString::number(lat,
'f', 15));
546 setText(QString::number(glat,
'f', 15));
548 setText(QString::number(lon,
'f', 15));
552 setText(QString::number(wlon,
'f', 15));
564 while(wlon < 0.0) wlon += 360.0;
569 setText(QString::number(lon,
'f', 15));
573 setText(QString::number(wlon,
'f', 15));
577 setText(QString::number(radius,
'f', 15));
588 setText(QString::number(projX,
'f', 15));
590 setText(QString::number(projY,
'f', 15));
596 int iMosaicOrigin = -1;
597 QString sSrcFileName =
"";
598 QString sSrcSerialNum =
"";
599 TrackMosaicOrigin(cvp, iline, isample, iMosaicOrigin, sSrcFileName, sSrcSerialNum);
601 setText(QString::number(iMosaicOrigin));
603 setText(QString(sSrcFileName));
605 setText(QString(sSrcSerialNum));
627 int piSample,
int &piOrigin, QString &psSrcFileName,
628 QString &psSrcSerialNum) {
641 trackingCube = cCube;
647 trackingCube->
read(trackingPortal);
649 unsigned int currentPixel = trackingPortal[0];
650 if (currentPixel != NULLUI4) {
655 psSrcFileName = trackingFileName.
name();
656 psSrcSerialNum = trackingTable.
pixelToSN(currentPixel);
657 piOrigin = trackingTable.
fileNameToIndex(trackingFileName, psSrcSerialNum);
661 else if(cCube->
hasTable(trackingTableName)) {
665 for(
int i = 0; i < cGrpBandBin.
keywords(); i++) {
667 for(
int j = 0; j < cKeyTrackBand.
size(); j++) {
668 if(cKeyTrackBand[j] ==
"TRACKING") {
675 if(iTrackBand > 0 && iTrackBand <= cCube->bandCount()) {
678 cOrgPortal.
SetPosition(piSample, piLine, iTrackBand + 1);
679 cCube->
read(cOrgPortal);
680 piOrigin = (int)cOrgPortal[0];
683 piOrigin -= VALID_MIN1;
687 piOrigin -= VALID_MIN2;
691 piOrigin -= FLOAT_MIN;
697 int iRecs = cFileTable.
Records();
698 if(piOrigin >= 0 && piOrigin < iRecs) {
699 psSrcFileName = QString(cFileTable[piOrigin][0]);
700 psSrcSerialNum = QString(cFileTable[piOrigin][1]);
712 if (piOrigin == -1) {
713 psSrcFileName =
"N/A";
714 psSrcSerialNum =
"N/A";
728 QVBoxLayout *dialogLayout =
new QVBoxLayout;
730 QLabel *dialogTitle =
new QLabel(
"<h3>Advanced Tracking Tool</h3>");
731 dialogLayout->addWidget(dialogTitle);
733 QTabWidget *tabArea =
new QTabWidget;
734 dialogLayout->addWidget(tabArea);
736 QScrollArea *recordTab =
new QScrollArea;
738 QVBoxLayout *recordLayout =
new QVBoxLayout;
739 recordContainer->setLayout(recordLayout);
740 QLabel *recordText =
new QLabel(
"To record, click on the viewport of interest and"
741 " press (r) while the mouse is hovering over the image.");
742 recordText->setWordWrap(
true);
743 recordLayout->addWidget(recordText);
744 recordTab->setWidget(recordContainer);
746 QScrollArea *helpTab =
new QScrollArea;
748 QVBoxLayout *helpLayout =
new QVBoxLayout;
749 helpContainer->setLayout(helpLayout);
750 QLabel *helpText =
new QLabel(
"In order to use <i>ctrl+c</i> to copy and <i>ctrl+v</i> to"
751 " paste, you need to double click on the cell you are copying"
752 " from (the text should be highlighted). Then double click on"
753 " the cell you are pasting to (you should see a cursor if the"
754 " cell is blank). When a cell is in this editing mode, most"
755 " keyboard shortcuts work.");
756 helpText->setWordWrap(
true);
757 recordText->setWordWrap(
true);
758 helpLayout->addWidget(helpText);
759 helpTab->setWidget(helpContainer);
761 tabArea->addTab(recordTab,
"Record");
762 tabArea->addTab(helpTab,
"Table Help");
764 QPushButton *okButton =
new QPushButton(
"OK");
765 dialogLayout->addStretch();
766 dialogLayout->addWidget(okButton);
768 connect(okButton, SIGNAL(clicked()),
helpDialog, SLOT(accept()));
789 QTableWidgetItem *item =
new QTableWidgetItem(
"");
796 QAbstractItemView::PositionAtBottom);
873 if (QApplication::applicationName() ==
"") {
875 "application name before using the Isis::MainWindow class. Window "
876 "state and geometry can not be saved and restored", _FILEINFO_);
879 FileName config(
FileName(
"$HOME/.Isis/" + QApplication::applicationName() +
"/").path() +
"/" +
880 "advancedTrackTool.config");
int cubeLines() const
Return the number of lines in the cube.
QString PixelToString(double d)
Takes a double pixel value and returns the name of the pixel type as a string.
void radii(Distance r[3]) const
Returns the radii of the body in km.
int SizeOf(Isis::PixelType pixelType)
Returns the number of bytes of the specified PixelType.
void instrumentPosition(double p[3]) const
Returns the spacecraft position in body-fixed frame km units.
double LocalRadius(double lat) const
This method returns the local radius in meters at the specified latitude position.
iTime time() const
Returns the ephemeris time in seconds which was used to obtain the spacecraft and sun positions.
Cube display widget for certain Isis MDI applications.
ShapeModel * shape() const
Return the shape.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
virtual QString fileName() const
Returns the opened cube's filename.
@ Io
A type of error that occurred when performing an actual I/O operation.
double FocalPlaneY() const
Contains Pvl Groups and Pvl Objects.
void setCurrentIndex(int currentIndex)
Sets the current index to currentIndex.
double UniversalRingLongitude()
This returns a universal ring longitude (clockwise in 0 to 360 domain).
QTableWidget * table() const
Returns the table.
Buffer for containing a two dimensional section of an image.
virtual double ObliquePixelResolution()
Returns the oblique pixel resolution at the current position in meters/pixel.
A single keyword-value pair.
This is free and unencumbered software released into the public domain.
virtual double UniversalLatitude()
This returns a universal latitude (planetocentric).
virtual bool SetImage(const double sample, const double line)
Sets the sample/line values of the image to get the lat/lon values.
QString name() const
Returns the name of the file excluding the path and the attributes in the file name.
Parse and return pieces of a time string.
void read(Blob &blob, const std::vector< PvlKeyword > keywords=std::vector< PvlKeyword >()) const
This method will read data from the specified Blob object.
File name manipulation and expansion.
Table readTable(const QString &name)
Read a Table from the cube.
double UndistortedFocalPlaneX() const
Gets the x-value in the undistorted focal plane coordinate system.
static QString Compose(Pvl &label, bool def2filename=false)
Compose a SerialNumber from a PVL.
virtual double Declination()
Returns the declination angle (sky latitude).
virtual double Longitude() const
This returns a longitude with correct longitude direction and domain as specified in the label object...
QString name() const
Gets the shape name.
ProjectionType projectionType() const
Returns an enum value for the projection type.
double grayPixel(int sample, int line)
Gets the gray pixel.
Container for cube-like labels.
CameraDistortionMap * DistortionMap()
Returns a pointer to the CameraDistortionMap object.
double UndistortedFocalPlaneZ() const
Gets the z-value in the undistorted focal plane coordinate system.
double LocalSolarTime()
Return the local solar time in hours.
virtual double RightAscension()
Returns the right ascension angle (sky longitude).
virtual Longitude solarLongitude()
Returns the solar longitude.
void addToTable(bool setOn, const QString &heading, const QString &menuText="", int insertAt=-1, Qt::Orientation o=Qt::Horizontal, QString toolTip="")
Adds a new column to the table when a new curve is added to the plot.
Distance measurement, usually in meters.
virtual Target * target() const
Returns a pointer to the target object.
void setTrackListItems(bool track=false)
If this property is true, the class will keep track of the checked/unchecked items in the dock area w...
void showTable()
This method checks to see if the table has been created.
void clearRow(int row)
This method clears the text of the given row.
double SpacecraftAzimuth()
Return the Spacecraft Azimuth.
int currentIndex() const
Returns the current index.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
Base class for Map Projections of plane shapes.
Contains multiple PvlContainers.
virtual double UniversalLongitude() const
Returns the positive east, 0-360 domain longitude, in degrees, at the surface intersection point in t...
bool hasGroup(const QString &group) const
Return if the cube has a specified group in the labels.
Base class for Map TProjections.
double redPixel(int sample, int line)
Gets the red pixel.
Cube * trackingCube() const
Class for storing Table blobs information.
bool isLinked() const
Is the viewport linked with other viewports.
void setCurrentRow(int row)
Sets the current row to row.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
Distort/undistort focal plane coordinates.
Table to store tracking information for a mosaic.
double NorthAzimuth()
Returns the North Azimuth.
IO Handler for Isis Cubes.
a subclass of the qisis mainwindow, tablemainwindow handles all of the table tasks.
static double To180Domain(const double lon)
This method converts a ring longitude into the -180 to 180 domain.
int cubeSamples() const
Return the number of samples in the cube.
FileName pixelToFileName(unsigned int pixel)
Returns the FileName that corresponds to a pixel value.
virtual int bandCount() const
Returns the number of virtual bands for the cube.
Defines an angle and provides unit conversions.
virtual bool SetWorld(const double x, const double y)
This method is used to set a world coordinate.
virtual double IncidenceAngle() const
Returns the incidence angle in degrees.
int fileNameToIndex(FileName file, QString serialNumber)
Returns the index of the filename/serialnumber combination.
static double To180Domain(const double lon)
This method converts a longitude into the -180 to 180 domain.
const double Null
Value for an Isis Null pixel.
CameraFocalPlaneMap * FocalPlaneMap()
Returns a pointer to the CameraFocalPlaneMap object.
@ Programmer
This error is for when a programmer made an API call that was illegal.
double FocalPlaneX() const
bool hasTable(const QString &name)
Check to see if the cube contains a pvl table by the provided name.
PixelType pixelType() const
Convert between distorted focal plane and detector coordinates.
bool IsValidPixel(const double d)
Returns if the input pixel is valid.
virtual double PixelResolution()
Returns the pixel resolution at the current position in meters/pixel.
void setStatusMessage(QString message)
sets the status message in the lower lefthand corner of the window.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
QString pixelToSN(unsigned int pixel)
Returns the serial number that corresponds to a pixel value.
virtual double UniversalLongitude()
This returns a universal longitude (positive east in 0 to 360 domain).
double meters() const
Get the distance in meters.
int size() const
Returns the number of values stored in this keyword.
double ToPlanetographic(const double lat) const
This method converts a planetocentric latitude to a planetographic latitude.
double degrees() const
Get the angle in units of Degrees.
virtual double PhaseAngle() const
Returns the phase angle in degrees.
int currentRow() const
Returns the current row.
@ Triaxial
These projections are used to map triaxial and irregular-shaped bodies.
void LocalPhotometricAngles(Angle &phase, Angle &incidence, Angle &emission, bool &success)
Calculates LOCAL photometric angles using the DEM (not ellipsoid).
int keywords() const
Returns the number of keywords contained in the PvlContainer.
void Coordinate(double p[3]) const
Returns the x,y,z of the surface intersection in BodyFixed km.
void viewportToCube(int x, int y, double &sample, double &line) const
Turns a viewport into a cube.
bool IsSky() const
Returns true if projection is sky and false if it is land.
Adds specific functionality to C++ strings.
virtual double EmissionAngle() const
Returns the emission angle in degrees.
int Records() const
Returns the number of records.
double YCoord() const
This returns the projection Y provided SetGround, SetCoordinate, SetUniversalGround,...
double UndistortedFocalPlaneY() const
Gets the y-value in the undistorted focal plane coordinate system.
void SetPosition(const double sample, const double line, const int band)
Sets the line and sample position of the buffer.
ProjectionType
This enum defines the subclasses of Projection supported in Isis.
double SunAzimuth()
Returns the Sun Azimuth.
double XCoord() const
This returns the projection X provided SetGround, SetCoordinate, SetUniversalGround,...
QString UTC(int precision=8) const
Returns the internally stored time, formatted as a UTC time.
QString path() const
Returns the path of the file name.
This is free and unencumbered software released into the public domain.
virtual double SlantDistance() const
Return the distance between the spacecraft and surface point in kmv.
Distance LocalRadius() const
Returns the local radius at the intersection point.
Projection * projection() const
double UniversalRingRadius()
This returns a universal radius, which is just the radius in meters.
virtual double UniversalLatitude() const
Returns the planetocentric latitude, in degrees, at the surface intersection point in the body fixed ...