File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
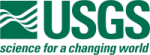 |
Isis 3 Programmer Reference
|
8 #include "EmbreeShapeModel.h"
15 #include "IException.h"
18 #include "Longitude.h"
19 #include "NaifStatus.h"
21 #include "ShapeModel.h"
32 EmbreeShapeModel::EmbreeShapeModel()
65 m_targetManager(targetManager),
95 QString msg =
"Cannot create a EmbreeShape from " +
m_shapeFile;
112 m_targetManager(targetManager),
113 m_tolerance(DBL_MAX),
114 m_shapeFile(shapefile) {
133 QString msg =
"Cannot create a EmbreeShape from " +
m_shapeFile;
162 std::vector<double> lookDirection) {
207 const std::vector<double> &observerPos,
208 const bool &backCheck) {
230 for (
int i = 0 ; i < hits.size() ; i++) {
236 obsRay.org[0] = observerPos[0];
237 obsRay.org[1] = observerPos[1];
238 obsRay.org[2] = observerPos[2];
239 obsRay.dir[0] = lookVector[0];
240 obsRay.dir[1] = lookVector[1];
241 obsRay.dir[2] = lookVector[2];
244 obsRay.instID = RTC_INVALID_GEOMETRY_ID;
245 obsRay.geomID = RTC_INVALID_GEOMETRY_ID;
246 obsRay.primID = RTC_INVALID_GEOMETRY_ID;
247 obsRay.mask = 0xFFFFFFFF;
285 const std::vector<double> &observerPos,
286 const bool &backCheck) {
316 for (
int i = 0 ; i < hits.size() ; i++) {
322 obsRay.org[0] = observerPos[0];
323 obsRay.org[1] = observerPos[1];
324 obsRay.org[2] = observerPos[2];
325 obsRay.dir[0] = lookVector[0];
326 obsRay.dir[1] = lookVector[1];
327 obsRay.dir[2] = lookVector[2];
330 obsRay.instID = RTC_INVALID_GEOMETRY_ID;
331 obsRay.geomID = RTC_INVALID_GEOMETRY_ID;
332 obsRay.primID = RTC_INVALID_GEOMETRY_ID;
333 obsRay.mask = 0xFFFFFFFF;
364 std::vector<double> intersectArray(3);
367 intersectArray.begin() );
449 const std::vector<double> lookDirection) {
465 std::vector<double> intersectVect(3);
503 QString mess =
"Intercept point does not exist - cannot provide normal vector";
548 QString msg =
"An intersection must be defined before computing the surface normal.";
553 QString msg =
"The surface point intersection must be valid to compute the surface normal.";
558 QString msg =
"A valid target must be defined before computing the surface normal.";
571 surfnm_c(radii[0].kilometers(), radii[1].kilometers(), radii[2].kilometers(),
598 std::vector<double> localNormal;
616 return ellipsoidEmission;
640 double latAngle = lat.
radians();
641 double lonAngle = lon.
radians();
642 ray.dir[0] = cos(latAngle) * cos(lonAngle);
643 ray.dir[1] = cos(latAngle) * sin(lonAngle);
644 ray.dir[2] = sin(latAngle);
669 ray.instID = RTC_INVALID_GEOMETRY_ID;
670 ray.geomID = RTC_INVALID_GEOMETRY_ID;
671 ray.primID = RTC_INVALID_GEOMETRY_ID;
672 ray.mask = 0xFFFFFFFF;
676 std::vector<double> surfVect(3);
684 ray.dir[0] = direction[0];
685 ray.dir[1] = direction[1];
686 ray.dir[2] = direction[2];
710 int hitCount = ray.
lastHit + 1;
714 for (
int i = 0 ; i < hitCount ; i++) {
double getTolerance() const
Get the tolerance used when checking if the stored surface point is visible.
double kilometers() const
Get the distance in kilometers.
RTCMultiHitRay latlonToRay(const Latitude &lat, const Longitude &lon) const
Given a latitude and longitude, create a ray that goes from the origin of the target through that lat...
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
virtual void clearSurfacePoint()
Clears or resets the current surface point.
int lastHit
Index of the last hit in the hit containers.
This class is designed to encapsulate the concept of a Latitude.
SurfacePoint * surfaceIntersection() const
Returns the surface intersection for this ShapeModel.
virtual void setSurfacePoint(const SurfacePoint &surfacePoint)
Set surface intersection point.
virtual bool isDEM() const
Indicates that this shape model is not from a DEM.
std::vector< Distance > targetRadii() const
Returns the radii of the body in km.
void setHasIntersection(bool b)
Sets the flag to indicate whether this ShapeModel has an intersection.
virtual double incidenceAngle(const std::vector< double > &uB)
Computes and returns incidence angle, in degrees, given the illuminator position.
virtual bool intersectSurface(std::vector< double > observerPos, std::vector< double > lookDirection)
This method computes an intercept point given an observer location and look direction using the Embre...
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
double maximumSceneDistance() const
Return the maximum distance within the scene.
virtual bool isVisibleFrom(const std::vector< double > observerPos, const std::vector< double > lookDirection)
Check if the current internalized surface point is visible from an observer position and look directi...
static double magnitude(const Vector &vector)
Computes the magnitude (i.e., the length) of the given vector using the Euclidean norm (L2 norm).
QVector< double > ellipsoidNormal()
Compute the true surface normal vector of an ellipsoid.
static Vector vector(double v0, double v1, double v2)
Constructs a 3 dimensional vector with the given component values.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
void setNormal(const std::vector< double >)
Sets the normal for the currect intersection point.
EmbreeTargetManager * m_targetManager
!< The target body and Embree objects for intersection.
Distance measurement, usually in meters.
This class is designed to encapsulate the concept of a Longitude.
virtual Distance localRadius(const Latitude &lat, const Longitude &lon)
Determine radius at a given lat/lon grid point.
@ Traverse
Search child objects.
@ Kilometers
The distance is being specified in kilometers.
Struct for capturing occluded plates when using embree::rtcintersectscene.
void setName(QString name)
Sets the shape name.
virtual void calculateSurfaceNormal()
Return the surface normal of the ellipsoid.
boost::numeric::ublas::vector< double > Vector
Definition for an Isis::LinearAlgebra::Vector of doubles.
Class for managing the construction and destruction of EmbreeTargetShapes.
Contains multiple PvlContainers.
virtual void calculateDefaultNormal()
Return the surface normal of the ellipsoid as the default.
Struct for capturing multiple intersections when using embree::rtcintersectscene.
virtual double incidenceAngle(const std::vector< double > &uB)
Computes and returns incidence angle, in degrees, given the illuminator position.
virtual ~EmbreeShapeModel()
Destructor that notifies the target shape manager that the target shape is no longer in use.
QVector< RayHitInformation > sortHits(RTCMultiHitRay &ray, LinearAlgebra::Vector &observer)
Sort all intersections by a ray based on distance to a point.
void updateIntersection(const RayHitInformation hitInfo)
Update the ShapeModel given an intersection and normal.
EmbreeTargetShape * create(const QString &shapeFile)
Get a pointer to an EmbreeTargetShape containing the information from a shape file.
void FromNaifArray(const double naifValues[3])
A naif array is a c-style array of size 3.
static Vector normalize(const Vector &vector)
Returns a unit vector that is codirectional with the given vector by dividing each component of the v...
virtual void clearSurfacePoint()
Flag that the ShapeModel does not have a surface point or normal.
double m_tolerance
!< This manages EmbreeTargetShapes to allow for sharing of them between EmbreeShapeModels and deletes...
virtual void calculateLocalNormal(QVector< double * > cornerNeighborPoints)
Compute the normal for a local region of surface points.
void setHasNormal(bool status)
Sets the flag to indicate whether this ShapeModel has a surface normal.
RayHitInformation getHitInformation(RTCMultiHitRay &ray, int hitIndex)
Extract the intersection point and unit surface normal from an RTCMultiHitRay that has been intersect...
unsigned ignorePrimID
IDs of the primitives (trinagles) which should be ignored.
bool hasNormal() const
Returns surface point normal status.
Distance GetLocalRadius() const
Return the radius of the surface point.
@ Programmer
This error is for when a programmer made an API call that was illegal.
bool isOccluded(RTCOcclusionRay &ray)
Check if a ray intersects the target body.
Namespace for the standard library.
bool hasValidTarget() const
Returns the status of the target.
RTCMultiHitRay pointToRay(const SurfacePoint &point) const
Given a surface point, create a ray that goes from the origin of the target to the surface point.
Define shapes and provide utilities for Isis targets.
void free(const QString &shapeFile)
Notify the manager that an EmbreeTargetShape is no longer in use.
void intersectRay(RTCMultiHitRay &ray)
Intersect a ray with the target shape.
EmbreeShapeModel()
Default constructor sets type to a TIN.
void setTolerance(const double &tolerance)
Set the tolerance used when checking if the stored surface point is visible.
void ToNaifArray(double naifOutput[3]) const
A naif array is a c-style array of size 3.
This is free and unencumbered software released into the public domain.
bool hasIntersection()
Returns intersection status.
This is free and unencumbered software released into the public domain.
QString m_shapeFile
!< Tolerance for checking visibility.
This class is used to create and store valid Isis targets.
This class defines a body-fixed surface point.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
std::vector< double > normal()
Returns the local surface normal at the current intersection point.
double radians() const
Convert an angle to a double.